Making Sense of HTML5
- 5/15/2013
- Elements of a webpage
- Collecting data
- Multimedia elements
- Summary
Broadly speaking, the short words are the best, and the old words best of all.
—Winston Churchill
HTML5 is the latest version of the HTML language—the popular text-based language used to define the content of webpages. HTML appeared on the scene in the early 1990s. In the beginning it was merely a markup language apt at describing simple documents. A markup language is a language based on a set of markers that wrap text and give it a special meaning.
Initially, the set of HTML markup elements, called “tags” or (better) “elements,” was fairly limited. It contained elements to define references to other documents and headings, to link to images and paragraphs, and apply basic text styling such as bold or italic. Over the years, however, the role of HTML grew beyond imagination, progressing from being a simple language that described documents to a language used to define the user interface of web applications. That trend continues today with HTML5.
The latest version of HTML5 removes some of the older elements and makes it easier to keep elements that provide style information in one place, and elements that provide text and define the layout of the text, in another place. As you’ll see in more detail in the next chapter, style information can be defined through a special distinct file known as a Cascading Style Sheet (CSS). In addition, HTML5 adds some new elements suitable for including multimedia content and drawing, and several new frameworks for manipulating the content of the page programmatically.
With HTML5 alone, you still won’t be able to go too far toward building a complete application. However, the union of HTML5, CSS, and JavaScript functions as a close approximation to a full programming language.
- You use HTML5 to define the layout of the user interface and to insert text and multimedia.
- You use CSS to add colors, style, and shiny finishes.
- Finally, you use JavaScript to add behavior by gluing together pieces of native frameworks such as Document Object Model (DOM), local storage, geolocation and, for example, all the specific services of Windows 8 exposed via the Windows 8 JavaScript library (WinJs). The DOM, in particular, is the collection of programmable objects that expose the structure of the current document to coders.
In the rest of this chapter, you’ll briefly explore the basics of the HTML5 markup elements, including input forms and multimedia. Neither this chapter nor the rest of the book covers every aspect of the basics of HTML. If you need a refresher on the fundamentals of HTML, you can refer to the book Start Here! Learn HTML5, by Faithe Wempen (Microsoft Press, 2012).
Elements of a webpage
HTML5 comes about a decade after its most recent predecessor (HTML4). Looking at what’s new in HTML5, one could reasonably say that all these years have not passed in vain. HTML5 provides a set of new elements that offer several native functionalities that developers and designers used to have to code via artifacts and ingenious combinations of existing elements. Here’s a quick look at what’s relevant to creating a webpage with HTML5.
Building the page layout with HTML5
In the beginning of the web, most pages were designed as a text documents—meaning that their content developed vertically on a single logical column. Over the years, page layout became more and more sophisticated. Today, two-column and three-column layouts are much more common. In two- and three-column layouts, you also often find headers and footers surrounding the logical columns. Figure 2-1 shows the difference between the layouts at a glance.
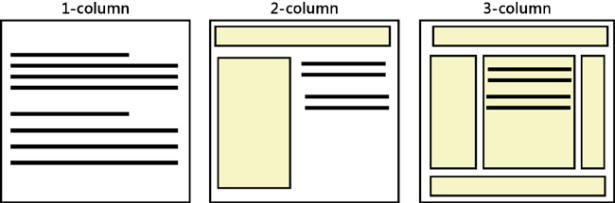
Figure 2-1 Different HTML page layouts.
Developers have been smart enough to build such complex layouts using basic HTML block elements such as DIV.
In HTML5, the multicolumn layout is recognized as a common layout and therefore gets full support via several new ad hoc markup elements.
Preparing the sample application
The examples you’ll be working with in this chapter are plain HTML pages showcasing some of the features available in HTML5 as supported in Internet Explorer 10. You won’t be creating an ad hoc Windows 8 application for each feature, but for this early example—to refresh what you saw in Chapter 1, “Using Visual Studio 2012 Express edition for Windows 8”—go ahead and create a container Windows 8 page that ties together all the links to the various standalone HTML5 pages.
Open up Visual Studio and create a new blank application. Name it Html5-Demos. When done, add the following code to the body of default.html so that it serves as the main menu for navigating into all of the sample HTML5 pages you’ll write throughout the chapter.
<body> <header> Start Here! Build <b>Windows 8</b> Applications with <b>HTML5</b> and <b>JavaScript</b> <hr /> HTML5 samples </header> <div id="links"> <a href="pages/multi.html">MULTI</a> <!-- Add here more links to HTML pages as we proceed in the chapter. --> </div> <footer> <hr /> Dino Esposito | Francesco Esposito </footer> </body>
Figure 2-2 shows the aspect of the resulting application. By clicking the links (such as, the MULTI link in the figure below) you force the operating system to open the webpage into Internet Explorer 10—the native browser in Windows 8.

Figure 2-2 The home page of the sample application for this chapter.
From now on, you’ll be creating plain HTML5 pages and adding an anchor tag <a> to the body of default.html.
From generic blocks to semantic elements
A large share of websites out there have a common layout that includes header and footer, as well as a navigation bar on the left of the page. More often than not, these results are achieved by using DIV elements styled to align to the left or the right.
Let’s add a new HTML page to the project: right-click the project node in Solution Explorer and choose Add | New Item from the subsequent flyout menu. What you get next is the window shown in Figure 2-3. From that window, you then choose a new HTML page and save it as multi.html.

Figure 2-3 Creating a new HTML page in Visual Studio.
Next, from within Visual Studio double-click the newly created HTML page and replace the content with the following markup.
<!DOCTYPE html> <html> <head> <title>MULTI-COLUMN LAYOUT</title> </head> <body> <a href="/default.html">Back</a> <hr /> <div id="page"> <div id="header"> Header of the page <hr /> </div> <div id="navigation-bar"> <ul> <li> Home </li> <li> Find us </li> <li> Job opportunities </li> </ul> </div> <div id="container"> <div id="left-sidebar"> Left sidebar <ul> <li> Article #1 </li> <li> Article #2 </li> <li> Article #3 </li> </ul> </div> <div id="content"> This is the main content of the page </div> <div id="right-sidebar"> Right sidebar </div> </div> <div id="footer"> <hr /> Footer of the page </div> </div> </body> </html>
The id attribute of the DIV elements are given self-explanatory names that help with understanding their intended role. Therefore, the HTML page includes header, navigation bar, footer, and a three-column layout in between the element named container. Figure 2-4 shows how multi.html renders on Internet Explorer.

Figure 2-4 The multi.html page as it is rendered by Internet Explorer 10.
The preceding markup alone, however, doesn’t produce the expected results and the page doesn’t really show any multicolumn layout. For that, you need to add ad hoc graphic styles to individual DIV elements to make them float and anchor to the left or right edge. You add graphic style to an HTML page using CSS markup, placed in a CSS file. The next chapter provides a quick summary of CSS. The real point of this demo is a little different.
As you can see, each DIV element is made distinguishable from others only by the name of the id attribute. Yet, each DIV element plays a clear role that makes it fairly different from others—header is different from footer, and both are different from left or right sidebars.
Header and footer elements
HTML5 brings a selection of new block elements with specific names and clear behavior. The set of new elements was determined by looking at the most common layouts used by page authors. For example, in HTML5 header and footer are new plain block elements you use to indicate a header and footer. Similar elements exist for most of the semantic elements in the previous listing. Here’s how you can rewrite the page multi.html using only HTML5-specific elements. Name this page multi5.html. The listing below shows the content of the body tag for the new page.
<header> Header of the page <hr /> </header> <nav> <a href="..."> Home </a> <a href="..."> Find us </a> <a href="..."> Job opportunities </a> </nav> <article> <aside> Left sidebar <ul> <li> Article #1 </li> <li> Article #2 </li> <li> Article #3 </li> </ul> </aside> <article> <h1>Article #1</h1> <hr /> <section> Introduction </section> <section> First section </section> <section> Second section </section> </article> <aside> Right sidebar </aside> </article> <footer> <hr /> Footer of the page </footer>
You can insert header and footer using specific elements with a very simple syntax, as below:
<header> Markup </header> <footer> Markup </footer>
It is interesting to notice that you can have multiple header and footer elements in a HTML5 page. The most common use is to give the page a header and footer. However, you should consider these elements as blocks meant to represent heading content of a page or a section of a page and footers.
Section and article elements
HTML5 defines two similar-looking elements to represent the content of a page. The <section> element is slightly more generic, as it is meant to delimit a logical section of a HTML page. A logical section can be the content of a tab in a page designed as a collection of tabs.
At the same time, a logical section can also be a portion of the main content being displayed in the page. In this case, the section element is likely embedded in an <article> element.
<article> <h1>Article #1</h1> <hr /> <section> Introduction </section> <section> First section </section> <section> Second section </section> </article>
The aside element
A lot of HTML pages display part of their content on columns that lie side by side horizontally. The <aside> element has been introduced in HTML5 to quickly identify some content that is related to the content being displayed all around. The syntax of the <aside> element is straightforward:
<aside> Markup </aside>
A very common scenario where you might want to take advantage of the<aside> element is to define a sidebar in an article element and, more in general, to create multicolumn layouts for the content of the page or sections of the page.
The nav element
The <nav> element indicates a special section of the page content—the section that contains major navigation links. It should be noted that not all links you can have in a HTML page must be defined within a <nav> element. The <nav> element is reserved only for the most relevant links, such as those you would place on the main page navigation menu.
The syntax of the <nav> element is fairly intuitive. It consists of a list of <a> anchor elements listed within the <nav> element:
<nav> <a href="..."> Home </a> <a href="..."> Find us </a> <a href="..."> Job opportunities </a> </nav>
The <nav> element plays an important role in HTML5 because it indicates the boundaries of the section of the page that contains navigation links. This allows special page readers—such as browsers for disabled users—to better understand the structure of the page and optionally skip some content.
Miscellany of other new elements
Semantic block elements represent the largest family of new elements in HTML5. As mentioned, semantic elements are important not so much for the effect they produce in the page but because they increase the readability of the page significantly for developers, software, and especially browsers for disabled users.
Semantic block elements alone do not produce significant changes in the way in which HTML5-compliant browsers render the page. For example, to color and position a sidebar (that is, the <aside> element) where you like you still need to resort to CSS settings. However, using semantic elements reduces the noise of having too many generic DIV block elements whose role and scope is not immediately clear.
In addition to semantic elements, HTML5 provides a few new elements with an embedded behavior that couldn’t be obtained in earlier versions of HTML without resorting to a combination of CSS, markup, and JavaScript. Let’s see a few examples.
The details element
Many times you have small pieces of content in a page that you want to show or hide on demand. A good example is the title of some news and its actual content. Sometimes you want to display only the title but want to leave users free from clicking to expand the content and hide it to gain more space.
Before HTML5, you had to code all of this manually using a bit of HTML, CSS, and JavaScript code. In HTML5, the entire logic is left to the browser and all you have to do is type the following in an HTML page.
<details open> <summary>This is the title</summary> <div> This is the text of the news and was initially kept hidden from view </div> </details>
The <details> element is interpreted by the browser and used to implement a collapsible panel. The open attribute indicates whether you want the content to be displayed initially or not. The <summary> child element indicates the text for the clickable placeholder, whereas the remaining content is hidden or shown on demand. Note that all parts of the <details> element can be further styled at will using CSS.
The mark element
HTML5 also adds the <mark> element as a way to highlight small portions of text as if you were using a highlighter on a paper sheet. Using the <mark> element is easy; all you do is wrap some text in the <mark> element, as shown below:
The <mark>DETAILS</mark> element is not supported by Internet Explorer 10.
The entire text is rendered with default settings except the text enclosed in the <mark> element. Most HTML5 browsers have default graphical settings for marked text. Most commonly, these settings entail a yellow background. Needless to say, graphical effects of the <mark> element can be changed at will via CSS.
Figure 2-5 shows how the previous text looks using Internet Explorer 10.
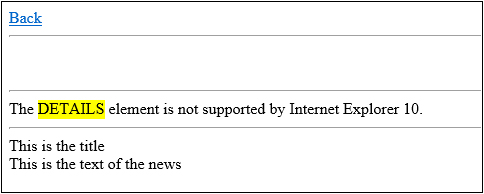
Figure 2-5 The mark element in action.
The dataList element
For a long time, HTML developers asked loudly for the ability to offer a list of predefined options for a text field. The use-case is easy to figure out. Imagine a user required to type the name of a city in a text field. As a page author, you want to leave the user free of entering any text; at the same time, though, you want to provide a few predefined options that can be selected and entered with a single click. Up until HTML5, this feature had to be coded via JavaScript, as HTML provided only two options natively: free text with no auto-completion or a fixed list of options with no chance of typing anything. The new <datalist> element fills the gap. Copy the following text to the body of a new HTML page named datalist.html.
<input list="cities" /> <datalist id="cities"> <option value="Rome"> <option value="New York"> <option value="London"> <option value="Paris"> </datalist>
In the example, the <datalist> element is bound to a particular input field—the input field named cities. It is interesting to notice that the binding takes place through a new attribute defined on the <input> element: the list attribute. The attribute gets the name of a <datalist> element to be used as the source of the input options.
When the input field gets the input focus, then the content of the <datalist> element is used to autocomplete what the user is typing. Figure 2-6 shows the element in action on Internet Explorer.

Figure 2-6 The datalist element in action.
Elements removed from older HTML versions
HTML5 adds some new elements, but also removes a few elements whose presence would only increase redundancy once combined with the new capabilities of CSS and new elements in HTML5.
The list of elements no longer supported most notably includes frame and font elements. It should be noted, though, that the <iframe> element remains available.
In addition, a few style elements such as <center>, <u>, and <big> are removed. The reason is that this functionality can be achieved easily through CSS. Probably due to the much larger use that page authors made over the years, HTML5 still supports elements such as <b> (for bold text) and <i> (for italic text) that are logically equivalent to the now unsupported <u> and <big> elements.