JavaScript Programming Basics
- 9/15/2012
- JavaScript Placement: Revisited
- Basic JavaScript Syntax
- JavaScript Variables and Data Types
- Looping and Conditionals in JavaScript
- Summary
After completing this chapter, you will be able to
Know where to place JavaScript in a webpage
Understand basic JavaScript syntax
Create JavaScript variables and understand common data types
Use looping and conditional constructs in your JavaScript code
NOW THAT YOU HAVE A TASTE of what JavaScript can do, it’s time to look at JavaScript’s inner workings. Admittedly, the chapter won’t get too far into the inner workings—just far enough to make you proficient but not overwhelmed. Between this chapter and the next, you’ll have a firm foundation with which you’ll be able to build programs and troubleshoot existing JavaScript programs.
In this chapter, you’ll look at the syntax and rules of the language. This includes things as simple as how to place a comment in a program to how to create a variable and perform conditional logic. This material is largely condensed while still providing what you need to know. Like Chapter 1 this chapter will also focus primarily on JavaScript and its use in web applications. However, the information applies to JavaScript for Microsoft Windows 8, as well.
JavaScript Placement: Revisited
You learned in Chapter 1 that JavaScript programs are placed within the <head> or <body> sections of a webpage. For external scripts such as jQuery, it’s common to place the reference in the <head> section, but there’s nothing preventing you from placing the reference to the external script in either the head or body of the page. This section expands on the placement of JavaScript by creating a base page and an external JavaScript file. The files created here will be used as the basis for examples throughout the book.
The basic HTML page that will be used should look familiar if you’ve just come from Chapter 1. In fact, this chapter will use the project created in Chapter 1—specifically the structured layout as shown in Chapter 1—with css and js folders created in the project or document root. See Figure 2-1 for an example of this structure.

Figure 2-1 The folder structure for development for the book should include css and js folders within the project.
Create a new file, or alter your existing index.html to match the code in the following sample. If you create a new file, save it as index.html (which you can find in the companion content as index.html).
<!DOCTYPE html> <html> <head> <title>Listing 1-1</title> <script type="text/javascript" src="js/external.js"></script> </head> <body> </body> </html>
With index.html created, it’s time to create an external JavaScript file. Within Microsoft Visual Studio, in Solution Explorer, right-click the js folder, click Add, and then click Add New Item. The Add New Item dialog box opens. Click JavaScript File and then in the Name text box, type external.js, as shown in Figure 2-2.
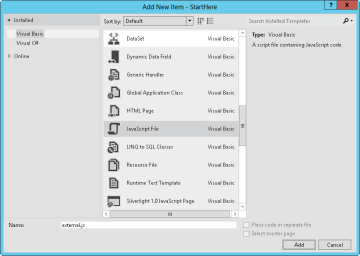
Figure 2-2 Creating a new JavaScript file.
Click Add and a new JavaScript file appears.
Some versions of Visual Studio don’t allow you to set the name of the file on creation, as in the previous example. If this is the case, the file will be named JavaScript1.js. However, the file referenced in the HTML file is external.js within the js folder. Therefore, the default JavaScript1.js file name will need to change.
Within Visual Studio, click File and then click Save As. The Save File As dialog box opens. Open the js folder and then enter external.js in the File Name text box, as shown in Figure 2-3. (Note that your screen shot might be slightly different than mine, and again, you only need to do this if your version of Visual Studio didn’t allow you to set the name when you added the file.)

Figure 2-3 Saving the external.js file into the js folder.
You now have a basic HTML page and an external JavaScript file. From here, the remainder of the chapter (and indeed the book) will use these files to show examples.