The Essential .NET Data Types
- 8/15/2011
- Numeric Data Types
- The Char Data Type
- The String Data Type
- The Boolean Data Type
- The Date Data Type
- .NET Equivalents of Base Data Types
- Constants and Read-Only Fields (Read-Only Members)
.NET Equivalents of Base Data Types
There is a .NET equivalent for each base data type in Visual Basic, as shown in Table 6-6.
Table 6-6 The Base Visual Basic Data Types and Their .NET Equivalents
Base data type in Visual Basic |
.NET data type equivalent |
Byte |
System.Byte |
SByte |
System.SByte |
Short |
System.Int16 |
UShort |
System.UInt16 |
Integer |
System.Int32 |
UInteger |
System.UInt32 |
Long |
System.Int64 |
ULong |
System.UInt64 |
Single |
System.Single |
Double |
System.Double |
Decimal |
System.Decimal |
Boolean |
System.Boolean |
Date |
System.DateTime |
Char |
System.Char |
String |
System.String |
Table 6-6 illustrates that it doesn’t matter at all whether you declare a 32-bit integer with
Dim loc32BitInteger as Integer
or with
Dim loc32BitInteger as System.Int32
The object variable loc32BitInteger ends up with the exact same type in both cases. Take a look at the IML-generated code that follows:
Public Shared Sub main() Dim locDate As Date = #12/14/2003# Dim locDate2 As DateTime = #12/14/2003 12:13:22 PM# If locDate > locDate2 Then Console.WriteLine("locDate is larger than locDate2") Else Console.WriteLine("locDate2 is larger than locDate") End If
The generated lines of code verify that this is true:
.method public static void main() cil managed { // Code size 75 (0x4b) .maxstack 2 .locals init ([0] valuetype [mscorlib]System.DateTime locDate, [1] valuetype [mscorlib]System.DateTime locDate2, [2] bool VB$CG$t_bool$S0) IL_0000: nop IL_0001: ldc.i8 0x8c58fec59f98000 IL_000a: newobj instance void [mscorlib]System.DateTime::.ctor(int64) IL_000f: nop IL_0010: stloc.0 IL_0011: ldc.i8 0x8c59052cd35dd00 IL_001a: newobj instance void [mscorlib]System.DateTime::.ctor(int64) IL_001f: nop IL_0020: stloc.1 IL_0021: ldloc.0 IL_0022: ldloc.1 . . .
As the code highlighted in bold shows, both local variables have been declared as System.DateTime type. Notice also that Date variables are represented internally as Long values).
The GUID Data Type
GUID is the abbreviation for Globally Unique Identifier. The term “Global” refers to the fact that even though two GUID generators aren’t aware of each other’s existence and are spatially separated from each other, they are highly unlikely to produce two identical identifiers.
GUIDs are 16-byte (128-bit) values, usually represented in the format {XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX}, where each “X”represents a hexadecimal number between 0 and F.
In .NET, the name of the data type (GUID) corresponds to its abbreviation.
GUIDs are generally used as primary keys in databases, because it is extremely improbable that two computer systems generate identical GUIDs. Database tables with foreign key identifiers based on GUIDs are particularly useful for synchronizing databases that cannot be constantly connected for technical reasons.
The Guid data type has a constructor with parameters that let you recreate an existing GUID via a string. Using it this way makes sense, for example, when a component (class, structure) that you have developed must always return the same unique identifier.
The following automatically implemented property of a class could therefore implement a UniqueID property:
Public Class AClass 'The property always returns the same GUID: Property UniqueID As Guid = New Guid("{46826D55-6FDD-44FA-BADE-515E04770816}") End Class
You can read more about classes and properties in Part II, “Object-Oriented Programming.”
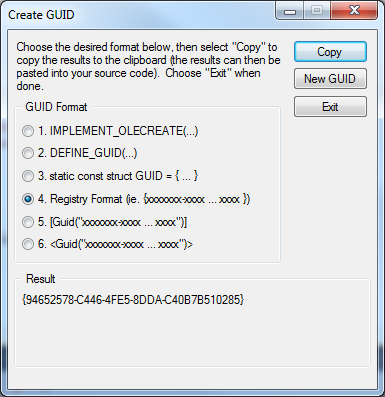
Figure 6-2 Using the Create GUID dialog box in the Visual Studio IDE, you can easily create a GUID constant.