Designing and Developing Windows Applications Using Microsoft .NET Framework 4: Designing the Presentation Layer
- 12/17/2011
- Objective 2.1: Choose the Appropriate Windows Technology
- Objective 2.2: Design the UI Layout and Structure
- Objective 2.3: Design Application Workflow
- Objective 2.4: Design Data Presentation and Input
- Objective 2.5: Design Presentation Behavior
- Objective 2.6: Design for UI Responsiveness
- Objective 2.6: Design for UI Responsiveness
- Chapter Summary
- Answers
The Presentation layer is the layer that directly interacts with the user. The user must be able to assimilate the data presented to him quickly and easily through the Presentation layer and also must be able to input data efficiently into the application. The Presentation layer itself is responsible for validating user input, maintaining responsiveness, and providing cues that enable an easy and accessible user experience. In this chapter, you will learn some of the important aspects of designing a Presentation layer. You will learn to choose between Windows Forms and Windows Presentation Foundation (WPF) for the technology used to build the Presentation layer, to design application layout and workflow, to handle data within the Presentation layer, and to develop a well-crafted and responsive user experience.
Objective 2.1: Choose the Appropriate Windows Technology
When creating applications for the desktop, today’s developer has two technologies to choose from: Windows Forms and WPF. Each technology provides its own set of advantages and drawbacks. In this section, you will learn the primary differences between the two technologies and how to decide which is most appropriate for your business situation.
Windows Forms
Windows Forms are the basis for most Microsoft Windows applications and can be configured to provide a variety of user interface (UI) options. The developer can create forms of various sizes and shapes and customize them to the user’s needs. Windows Forms is the older of the two Windows development technologies currently supported by Microsoft, and many skilled developers are available for creating and maintaining Windows Forms projects.
Windows Forms are the basic building blocks of the UI. They provide a container that hosts controls and menus and allow you to present an application in a familiar and consistent fashion. Forms can receive user input in the form of keystrokes or mouse interactions and can display data to the user through hosted controls. Most applications that require sustained user interaction will include at least one Windows Form, and complex applications frequently require several forms to allow the program to execute in a consistent and logical fashion.
Windows Forms have no inherent support for changing styles. Thus, if the look and feel of the application must change according to conditions on a particular client desktop, considerable coding will be required.
However, Windows Forms do provide excellent support for localization and globalization. You can create applications easily that display alternate strings and images based on the locations of deployment.
Navigation in Windows Forms typically involves switching between multiple individual forms. While this allows for parts of the application to be parceled out and presented to the user as discrete functional units, it can also create a disjointed kind of user experience. A more cohesive user experience is possible with Windows Forms using Multiple Document Interface (MDI) Forms.
WPF
WPF is the successor to Windows Forms for desktop application development. WPF applications differ from traditional Windows Forms applications in several ways. The most notable of these is that the code for the UI is separate from the code for application functionality. Although the code for the functionality of a project can be defined using familiar languages such as Microsoft Visual Basic .NET or Microsoft Visual C#, the UI of a WPF project typically is defined using a relatively new declarative syntax called Extensible Application Markup Language (XAML).
Three basic types of applications can be created with WPF:
Windows applications The most similar to Windows Forms applications. Windows applications are Windows-driven and provide a user experience that is familiar to Windows users and developers alike. Multiple windows can be open at any given time, and there is no built-in sense of navigation or history.
Navigation applications Provide a page-based user experience, similar to the experience of using a website. Typically, only a single page can be open at any given time, and the journal functionality keeps a record of pages visited and allows back-and-forth navigation. Unlike a website, however, a Navigation application is a compiled application that runs on your desktop computer and, like a Windows application, has full access to the resources of your computer.
XAML Browser Applications (XBAPs) Similar to Navigation applications, but they are designed to run in Windows Internet Explorer. These applications can be deployed to a server or to a website and are downloaded when instantiated. Applications of this type do not have full access to a computer’s resources. XBAPs run under a partial-trust environment, and resources such as the file system and the registry are inaccessible by XBAPs.
The choice of an application type depends upon several factors, the two most important of which are user experience and application requirements.
User experience Determines whether you choose a Windows application or a page-based application. For a user experience that most closely resembles a traditional Windows Forms application, a Windows application is the best choice. This application type allows you to create a menu-driven, multiwindow application that combines the rich functionality of a desktop application with the rich user experience that WPF provides. For a user experience that more closely resembles a website, you should choose a page-based application. Navigation applications and XBAPs provide built-in navigational functionality that allows you to structure the application paralleling a task, such as in an Internet shopping application or a wizard.
Application requirements If an application requires access to system resources that fall outside the Internet security zone, then an XBAP is not a good choice—a better choice would be a Windows application or a Navigation application. On the other hand, XBAPs allow you to deploy the application to a web server and have users start it from a hyperlink, thus making it easily accessible to a large-scale audience. If your application does not require access to system resources, an XBAP might be a good choice.
All types of WPF applications provide built-in support for changing styles and themes. Thus, if you want your application to respond to the style or theme of the desktop, it is a fairly simple matter to incorporate that functionality.
WPF applications have good support for localization and globalization, but support for this functionality is not as built-in as it is for Windows Forms. Thus, localizing an extensive WPF application will take more time and developer resources than a similarly scoped Windows Forms application.
Choosing Between Windows Forms and WPF
When choosing a technology for a client application, you must take into account several considerations. What are the skills of your developer force? Must the application be localized? What kind of support for styles and themes is needed? What sort of navigational experience is required? The following table illustrates the relative strengths of Windows Forms and WPF to help you make that decision.
Table 2-1 Important Properties of the Style Class
Criterion |
Windows Forms |
WPF |
Adoption among the developer community |
Strong |
Growing |
Support for localization and globalization |
Excellent |
Fair |
Support for changing styles and themes |
No |
Excellent |
Support for a traditional Windows-based client application |
Yes |
Yes |
Support for navigation through a page-based interface |
No |
Yes, through WPF Navigation applications |
Support for navigation through a multiple document interface (MDI application) |
Yes |
No |
Interoperating Between Windows Forms and WPF
In some cases, you might want to use WPF elements in a Windows Forms application, or Windows Forms elements in a WPF application. You can use the built-in interoperation functionality of the Microsoft .NET Framework easily to incorporate these elements as you choose.
Incorporating WPF Elements into a Windows Forms Application
You might want to incorporate WPF elements into your existing Windows Forms application (for example, a user control developed using WPF that takes advantage of specialized WPF behaviors in what is otherwise a Windows Forms application). You can add preexisting WPF user controls to your Windows Forms project by using the ElementHost control. As the name implies, the ElementHost control hosts a WPF element.
The most important property of ElementHost is the Child property. The Child property indicates the type of WPF control to be hosted by the ElementHost control. If the WPF control to be hosted is in a project that is a member of the solution, you can set the Child property in the Property Grid. Otherwise, the Child property must be set to an instance of the WPF control in code, as shown here:
Sample of Visual Basic.NET Code
Dim aWPFcontrol As New WPFProject.UserControl1 ElementHost1.Child = aWPFcontrol
Sample of C# Code
WPFProject.UserControl1 aWPFcontrol = new WPFProject.UserControl1; ElementHost1.Child = aWPFcontrol;
Some of the ambient properties of Windows Forms controls have WPF equivalents. These ambient properties are propagated to the hosted WPF controls and exposed as public properties on the ElementHost control. The ElementHost control translates each Windows Forms ambient property to its WPF equivalent. For more information, see Windows Forms and WPF Property Mapping at http://msdn.microsoft.com/library/ms751565.aspx in the online MSDN library.
Incorporating Windows Forms Elements in a WPF Application
Although WPF provides a wide variety of useful controls and features, you might find that some familiar functionality that you used in Windows Forms programming is not available. Notably absent are controls such as MaskedTextBox and PropertyGrid, as well as simple dialog boxes. Fortunately, you still can use many Windows Forms controls in your WPF applications.
Using Dialog Boxes in WPF Applications
Dialog boxes are one of the most notable things missing from the WPF menagerie of controls and elements. Because dialog boxes are separate UIs, however, they are relatively easy to incorporate into your WPF applications.
File Dialog Boxes
The file dialog box classes, OpenFileDialog and SaveFileDialog, are components that you want to use frequently in your applications. They allow you to browse the file system and return the path to the selected file. The OpenFileDialog and SaveFileDialog classes are very similar and share most important members. Important properties of the file dialog boxes are shown in Table 2-2, and important methods are shown in Table 2-3.
Table 2-2 Important Properties of the File Dialog Boxes
Property |
Description |
AddExtension |
Gets or sets a value indicating whether the dialog box automatically adds an extension to a file name if the user omits the extension. |
CheckFileExists |
Gets or sets a value indicating whether the dialog box displays a warning if the user specifies a file name that does not exist. |
CheckPathExists |
Gets or sets a value indicating whether the dialog box displays a warning if the user specifies a path that does not exist. |
CreatePrompt |
Gets or sets a value indicating whether the dialog box prompts the user for permission to create a file if the user specifies a file that does not exist. Available only in SaveFileDialog. |
FileName |
Gets or sets a string containing the file name selected in the file dialog box. |
FileNames |
Gets the file names of all selected files in the dialog box. Although this member exists for both the SaveFileDialog and the OpenFileDialog classes, it is relevant only to the OpenFileDialog class because it is possible to select more than one file only in OpenFileDialog. |
Filter |
Gets or sets the current file name filter string, which determines the choices that appear in the Save As File Type or Files Of Type box in the dialog box. |
InitialDirectory |
Gets or sets the initial directory displayed by the file dialog box. |
Multiselect |
Gets or sets a value indicating whether the dialog box allows multiple files to be selected. Available only in OpenFileDialog. |
OverwritePrompt |
Gets or sets a value indicating whether the Save As dialog box displays a warning if the user specifies a file name that already exists. Available only in SaveFileDialog. |
ValidateNames |
Gets or sets a value indicating whether the dialog box accepts only valid Win32 file names. |
Table 2-3 Important Methods of the File Dialog Boxes
Method |
Description |
OpenFile |
Opens the selected file as a System.IO.Stream object. For OpenFileDialog objects, it opens a read-only stream. For SaveFileDialog objects, it saves a new copy of the indicated file and then opens it as a read-write stream. You need to be careful when using the SaveFileDialog.OpenFile method to keep from overwriting preexisting files of the same name. |
ShowDialog |
Shows the dialog box modally, thereby halting application execution until the dialog box has been closed. Returns a DialogResult. |
To use a file dialog box in a WPF application, follow these steps:
In Solution Explorer, right-click the project name and choose Add Reference.
The Add Reference dialog box opens.
On the .NET tab, select System.Windows.Forms, and then click OK.
In code, create a new instance of the desired file dialog box, as shown here:
Sample of Visual Basic Code
Dim aDialog As New System.Windows.Forms.OpenFileDialog()
Sample of C# Code
System.Windows.Forms.OpenFileDialog aDialog = new System.Windows.Forms.OpenFileDialog();
Use the ShowDialog method to show the dialog box modally. After the dialog box is shown, you can retrieve the file name that was selected from the FileNames property. An example is shown here:
Sample of Visual Basic Code
Dim aResult As System.Windows.Forms.DialogResult aResult = aDialog.ShowDialog() If aResult = System.Windows.Forms.DialogResult.OK Then ' Shows the path to the selected file MessageBox.Show(aDialog.FileName) End If Sample of C# Code System.Windows.Forms.DialogResult aResult; aResult = aDialog.ShowDialog(); if (aResult == System.Windows.Forms.DialogResult.OK) { // Shows the path to the selected file MessageBox.Show(aDialog.FileName); }
WindowsFormsHost
While using dialog boxes in WPF applications is fairly straightforward, using controls is a bit more difficult. Fortunately, WPF provides an element specifically designed to ease this task, which is called WindowsFormsHost.
WindowsFormsHost is a WPF element that is capable of hosting a single child element that is a Windows Forms control. The hosted Windows Forms control automatically sizes itself to the size of the WindowsFormsHost. You can use the WindowsFormsHost to create instances of Windows Forms controls declaratively, and you also can set properties on hosted Windows Forms declaratively.
Adding a Windows Forms Control to a WPF Application
To use the WindowsFormsHost element in your WPF applications, first you must add to the XAML view a reference to the System.Windows.Forms.Integration namespace in the WindowsFormsIntegration assembly, as shown here. (This line has been formatted as two lines to fit on the printed page, but it should be on a single line in your XAML.)
xmlns:my="clr-namespace:System.Windows.Forms.Integration; assembly=WindowsFormsIntegration"
If you drag a WindowsFormsHost from the Toolbox to the designer, this reference is added automatically. You also must add a reference to the System.Windows.Forms namespace, as shown here:
xmlns:wf="clr-namespace:System.Windows.Forms;assembly=System.Windows.Forms"
Then you can create an instance of the desired Windows Forms control as a child element of a WindowsFormsHost element, as shown here:
<my:WindowsFormsHost Margin="48,106,30,56" Name="windowsFormsHost1"> <wf:Button Text="Windows Forms Button" /> </my:WindowsFormsHost>
Setting Properties of Windows Forms Controls in a WPF Application
You can set properties on a hosted Windows Forms control declaratively in XAML as you would any WPF element, as shown in bold here:
<my:WindowsFormsHost Margin="48,106,30,56" Name="windowsFormsHost1"> <wf:Button Text="Windows Forms Button" /> </my:WindowsFormsHost>
Although you can set properties declaratively on a hosted Windows Forms control, some of those properties will not have any meaning. For example, properties dealing with layout, such as Anchor, Dock, Top, and Left, have no effect on the position of the Windows Forms control. This is because its container is the WindowsFormsHost and the Windows Forms control occupies the entire interior of that element. To manage layout for a hosted Windows Forms control, you should set the layout properties of the WindowsFormsHost, as highlighted in bold here:
<my:WindowsFormsHost Margin="48,106,30,56" Name="windowsFormsHost1"> <wf:Button Text="Windows Forms Button" /> </my:WindowsFormsHost>
Setting Event Handlers on Windows Forms Controls in a WPF Application
Similarly, you can set event handlers declaratively in XAML, as shown in bold in the following example:
<my:WindowsFormsHost Margin="48,106,30,56" Name="windowsFormsHost1"> <wf:Button Click="Button_Click" Name="Button1" /> </my:WindowsFormsHost>
Note that events raised by Windows Forms controls are regular .NET events, not routed events, and therefore they must be handled at the source.
Obtaining a Reference to a Hosted Windows Forms Control in Code
In most cases, using simple declarative syntax with hosted Windows Forms controls is not sufficient—you have to use code to manipulate hosted Windows Forms controls. Although you can set the Name property of a hosted Windows Forms control, that name does not give you a code reference to the control. Instead, you must obtain a reference by using the WindowsFormsHost.Child property and casting it to the correct type. The following code example demonstrates how to obtain a reference to a hosted Windows Forms Button control:
Sample of Visual Basic Code
Dim aButton As System.Windows.Forms.Button aButton = CType(windowsFormsHost1.Child, System.Windows.Forms.Button)
Sample of C# Code
System.Windows.Forms.Button aButton; aButton = (System.Windows.Forms.Button)windowsFormsHost1.Child;
Choosing a Presentation Pattern
A presentation pattern separates the UI from its behavior and state. Compared to a traditional three-layer architecture, this results in separating the Presentation layer code from the Business Logic and Data code. Using a presentation pattern makes your code easier to maintain and test. Perhaps most important, it allows you to replace or extend the UI easily.
You certainly can develop a WPF or Windows Forms application without using a presentation pattern; that is exactly what Windows developers have been doing for most of the time Windows has existed. In fact, for a simple “Hello, world” application, using a presentation pattern would increase the complexity and decrease the code readability. When you create more complex enterprise applications that need to be tested and maintained, using a presentation pattern can reduce development costs greatly over time.
For .NET Framework applications, you can choose from three different presentation patterns:
Model-View-Controller (MVC) Designed for web applications, this model uses views that consist of HTML and .NET Framework code to create the UI by formatting and displaying data from the model. The model stores the data and interacts with the database. The Controllers process requests and user input.
Model-View-ViewModel (M-V-VM) Designed for WPF applications, this model closely resembles the MVC model. However, this model uses views that consist of XAML code to create the UI. The ViewModel is an abstraction of the view; it resides between the model and the view to present the model in a way that more closely resembles how the user will see it (thus reducing the amount of code required in the view itself). Views bind to the data they display by specifying the ViewModel as their DataContext, and views send data to the ViewModel using Commands that typically execute a ViewModel method.
Model-View-Presenter (MVP) Designed for WPF applications, this model closely resembles both M-V-VM and MVC. The user interacts with the view, and the view raises events that the presenter responds to. The presenter interacts with the model and updates the values shown in the view.
Figure 2-1 visually compares the three architectures. In particular, notice that the user interacts solely with the controller in the MVC architecture, and then the view collects information from the controller about the user’s request and retrieves data directly from the model. In the M-V-VM and MVP models, the user interacts directly with the view, and the ViewModel or presenter communicates with the model to prepare the data for the view.
Currently, MVC is the best choice for web applications. If you are creating a WPF application, you can choose either M-V-VM or MVP.
The key difference between M-V-VM and MVP is the way the ViewModel/presenter connects to the view. As illustrated by the directions of the arrows in Figure 2-1, this relationship is one-way for M-V-VM and two-way for MVP. Therefore, the M-V-VM ViewModel is loosely coupled with the view, whereas the MVP presenter is tightly coupled to the view.
To clarify, with the MVP model, the presenter needs a reference to the view because the presenter is responsible for manipulating the state of the view. With the M-V-VM model, the ViewModel is completely unaware that the view exists. With M-V-VM, the view sets a ViewModel as its DataContext and binds to properties on the ViewModel. Any changes to values in the ViewModel are reflected automatically on the view through that binding.
While the M-V-VM and MVP presentation patterns are very similar, M-V-VM is specialized to simplify WPF and Microsoft Silverlight development. Their structure is very similar, but there is simply better support for M-V-VM than for MVP. Therefore, M-V-VM is the best choice for WPF and Silverlight applications.
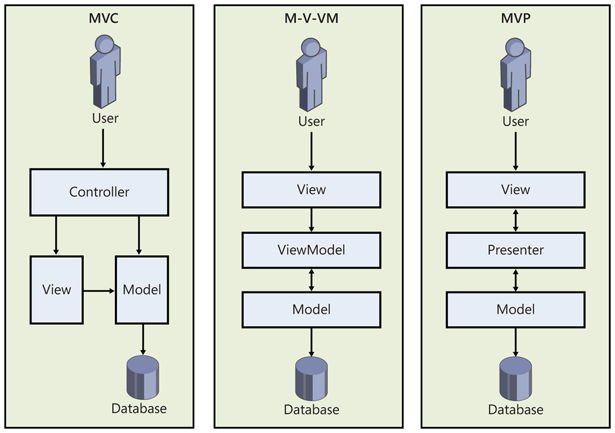
Figure 2-1 The three .NET Framework presentation patterns
Objective Summary
Windows Forms is a well-known technology that has a host of developers for development projects and provides excellent support for globalization and localization. However, it is not as good for changing styles, and it has no inherent navigation capability.
WPF is a relatively new technology with very powerful style and navigation features. However, because the technology is fairly new, it has not been adopted by as many developers, and support for globalization and localization is not as good as for Windows Forms.
Interoperability between WPF and Windows Forms is possible through the ElementHost and WindowsFormsHost controls.
Objective Review
Answer the following questions to test your knowledge of the information in this objective. You can find the answers to these questions and explanations of why each answer choice is correct or incorrect in the “Answers” section at the end of the chapter.
What class allows you to host a WPF control in a Windows Forms application?
WindowsFormsHost
ElementHost
Grid
Form
You are designing a Presentation layer for an application. You want this application to be responsive to changes in the style of the desktop so that it blends seamlessly with the appearance of the desktop, and you need this application to collect a variety of specially formed data via the MaskedTextBox control. What is the best development strategy for your Presentation layer?
Build using Windows Forms.
Build using WPF.
Build using Windows Forms that incorporate WPF controls.
Build using WPF and incorporate Windows Forms controls.