Understanding values and references
- By John Sharp
- 5/1/2022
- Copying value type variables and classes
- Understanding null values and nullable types
- Using ref and out parameters
- How computer memory is organized
- Casting data safely
- Summary
- Quick reference
In this sample chapter from Microsoft Visual C# Step by Step, 10th Edition, you will learn how the characteristics of the primitive types such as int, double, and char differ from the characteristics of class types.
After completing this chapter, you will be able to:
Explain the differences between a value type and a reference type.
Understand null values and how nullable types work.
Modify how arguments are passed as method parameters by using the ref and out keywords.
Describe how computer memory is organized to support value types and reference types.
Convert a value into a reference by using boxing.
Convert a reference back to a value by using unboxing and casting.
Chapter 7, “Creating and managing classes and objects,” demonstrated how to declare your own classes and how to create objects by using the new keyword. That chapter also showed you how to initialize an object by using a constructor. In this chapter, you’ll learn how the characteristics of the primitive types such as int, double, and char differ from the characteristics of class types.
Copying value type variables and classes
Most of the primitive types built into C#, such as int, float, double, and char (but not string, for reasons that will be covered shortly), are collectively called value types. These types have a fixed size, and when you declare a variable as a value type, the compiler generates code that allocates a block of memory big enough to hold a corresponding value. For example, declaring an int variable causes the compiler to allocate 4 bytes of memory (32 bits) to hold the integer value. A statement that assigns a value (such as 42) to the int causes the value to be copied into this block of memory.
Class types such as Circle (described in Chapter 7) are handled differently. When you declare a Circle variable, the compiler does not generate code that allocates a block of memory big enough to hold a Circle object. All it does is allot a small piece of memory that can potentially hold the address of (or a reference to) another block of memory containing a Circle object. (An address specifies the location of an item in memory.) The memory for the actual Circle object is allocated only when the new keyword is used to create the object.
A class is an example of a reference type. Reference types hold references to blocks of memory. To write effective C# code, you must understand the difference between value types and reference types.
Consider a situation in which you declare a variable named i as an int and assign it the value 42. If you declare another variable called copyi as an int and then assign i to copyi, copyi will hold the same value as i (42). However, even though copyi and i happen to hold the same value, two blocks of memory contain the value 42: one block for i and the other block for copyi. If you modify the value of i, the value of copyi does not change. Let’s see this in code:
int i = 42; // declare and initialize i int copyi = i; /* copyi contains a copy of the data in i: i and copyi both contain the value 42 */ i++; /* incrementing i has no effect on copyi; i now contains 43, but copyi still contains 42 */
The effect of declaring a variable c as a class type, such as Circle, is very different. When you declare c as a Circle, c can refer to a Circle object; the actual value held by c is the address of a Circle object in memory. If you declare an additional variable named refc (also as a Circle object) and you assign c to refc, refc will have a copy of the same address as c. In other words, there’s only one Circle object, and both refc and c now refer to it. Here’s an example in code:
var c = new Circle(42); Circle refc = c;
The following illustration shows both examples. The at sign (@) in the Circle objects represents a reference holding an address in memory.
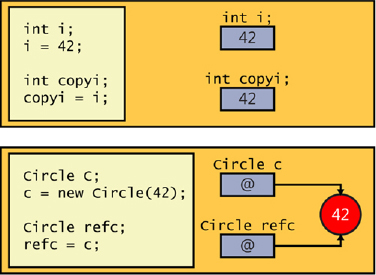
This difference is very important. It means that the behavior of method parameters depends on whether they are value types or reference types. You’ll explore this difference in the next exercise.
To use value parameters and reference parameters
Start Microsoft Visual Studio 2022, if it is not already running.
Open the Parameters solution, which is located in the \Microsoft Press\VCSBS\Chapter 8\Parameters folder in your Documents folder.
The project contains three C# code files: Pass.cs, Program.cs, and WrappedInt.cs.
Display the Pass.cs file in the Code and Text Editor window.
This file defines a class called Pass that is currently empty apart from a // TODO: comment.
Add a public static method called Value to the Pass class, replacing the // TODO: comment. This method should accept a single int parameter (a value type) called param and have the return type void. The body of the Value method should simply assign the value 42 to param, as shown in bold type in the following code example:
namespace Parameters { class Pass { public static void Value(int param) { param = 42; } } }
Display the Program.cs file in the Code and Text Editor window and then locate the doWork method of the Program class.
The doWork method is called by the Main method when the program starts running. As explained in Chapter 7, the method call is wrapped in a try block and followed by a catch handler.
Add four statements to the doWork method to perform the following tasks:
Declare a local int variable called i and initialize it to 0.
Write the value of i to the console by using Console.WriteLine.
Call Pass.Value, passing i as an argument.
Write the value of i to the console again.
By running Console.WriteLine before and after the call to Pass.Value, you can see whether the Pass.Value method actually modifies the value of i. The completed doWork method should look exactly like this:
static void doWork() { int i = 0; Console.WriteLine(i); Pass.Value(i); Console.WriteLine(i); }
On the Debug menu, select Start Without Debugging to build and run the program.
Confirm that the value 0 is written to the console window twice.
The assignment statement inside the Pass.Value method that updates the parameter and sets it to 42 uses a copy of the argument passed in, and the original argument i is completely unaffected.
Press Enter to close the application.
You’ll now see what happens when you pass an int parameter that’s wrapped within a class.
Display the WrappedInt.cs file in the Code and Text Editor window. This file contains the WrappedInt class, which is empty apart from a // TODO: comment.
Add a public instance field called Number of type int to the WrappedInt class, as shown in bold type in the following code:
namespace Parameters { class WrappedInt { public int Number; } }
Display the Pass.cs file in the Code and Text Editor window. Add a public static method called Reference to the Pass class. This method should accept a single WrappedInt parameter called param and have the return type void. The body of the Reference method should assign 42 to param.Number, as shown here:
public static void Reference(WrappedInt param) { param.Number = 42; }
Display the Program.cs file in the Code and Text Editor window. Comment out the existing code in the doWork method and add four more statements to perform the following tasks:
Declare a local WrappedInt variable called wi and initialize it to a new WrappedInt object by calling the default constructor.
Write the value of wi.Number to the console.
Call the Pass.Reference method, passing wi as an argument.
Write the value of wi.Number to the console again.
As before, with the calls to Console.WriteLine, you can see whether the call to Pass.Reference modifies the value of wi.Number. The doWork method should now look exactly like this (the new statements are in bold):
static void doWork() { // int i = 0; // Console.WriteLine(i); // Pass.Value(i); // Console.WriteLine(i); var wi = new WrappedInt(); Console.WriteLine(wi.Number); Pass.Reference(wi); Console.WriteLine(wi.Number); }
On the Debug menu, select Start Without Debugging to build and run the application.
This time, the two values displayed in the console window correspond to the value of wi.Number before and after the call to the Pass.Reference method. You should see that the values 0 and 42 are displayed.
Press Enter to close the application and return to Visual Studio 2022.
To explain what the previous exercise shows, the value of wi.Number is initialized to 0 by the compiler-generated default constructor. The wi variable contains a reference to the newly created WrappedInt object (which contains an int). The wi variable is then copied as an argument to the Pass.Reference method. Because WrappedInt is a class (a reference type), wi and param both refer to the same WrappedInt object. Any changes made to the contents of the object through the param variable in the Pass.Reference method are visible by using the wi variable when the method completes. The following diagram illustrates what happens when a WrappedInt object is passed as an argument to the Pass.Reference method.