JavaScript Programming Basics
- 9/15/2012
- JavaScript Placement: Revisited
- Basic JavaScript Syntax
- JavaScript Variables and Data Types
- Looping and Conditionals in JavaScript
- Summary
Looping and Conditionals in JavaScript
In a programming language, loops enable developers to perform some action repeatedly, to execute code a certain number of times, or to iterate through a list. For example, you might have a list of links on a webpage that need to have a certain style applied to them. Rather than program each change individually, you could iterate (loop) through the links and apply the style to each link within the loop code. This section looks at the common ways for looping or performing iterations in JavaScript.
Conditionals are statements that look for the “truthiness” of a given expression. For example, is the number 4 greater than the number 2? If so, do something interesting. A conditional is another way of expressing that same concept programmatically. If a certain condition is met, your program will do something. There’s also a condition for handling the situation when the specified condition isn’t met, known as the else condition. Conditionals in this form and related conditionals are also examined in this section.
Loops in JavaScript
Loops enable actions to be performed multiple times. In JavaScript (or any programming language), loops are commonly used to iterate through a collection such as an array and perform some action on each element therein.
The for loop is a primary construct for performing loop operations in JavaScript, as in this example:
var treeArray = ['Pine','Birch','Maple','Oak','Elm']; for (var i = 0; i < treeArray.length; i++) { alert("Tree is: " + treeArray[i]); }
In this example, each element in the array treeArray gets displayed through an alert() dialog. The code first instantiates a counter variable (i) and initializes it to 0, which (conveniently enough) is also the first index of a normal array. Next the counter variable i is compared to the length of the treeArray. Because i is 0 and the length of the treeArray is 5, the code within the braces will be executed. When the code within the braces completes, the counter variable, i, is incremented (thanks to the i++ within the for loop construct) and the whole process begins again—but this time, the counter variable is 1. Again, it’s compared to the length of the treeArray, which is still 5, so the code continues. When the counter variable reaches 5, the loop will end.
A similar looping construct available in JavaScript is the while loop. With a while loop, the programmer has more flexibility because the test condition that’s used can be changed by the programmer within the loop itself. Here’s the previous example written using a while loop. Note the need to manually increment the counter within the loop:
var treeArray = ['Pine','Birch','Maple','Oak','Elm']; var i = 0; while (i < treeArray.length) { alert("Tree is: " + treeArray[i]); i++; }
There is also a foreach statement available in JavaScript. However, as of this writing the foreach statement doesn’t work in many older browsers. Therefore, this book will concentrate on the more common and widely supported for loop in most places.
Additionally, you can get a slight speed improvement by setting the array length to its own variable outside of the loop construct, in this manner:
var treeArray = ['Pine','Birch','Maple','Oak','Elm']; var treeArrayLength = treeArray.length; for (var i = 0; i < treeArrayLength; i++) { alert("Tree is: " + treeArray[i]); }
This change means that the code doesn’t need to examine the length of the treeArray for every iteration through the loop; instead, the length is examined once, before the loop starts.
Conditionals in JavaScript
Conditionals are tests to determine what should happen when a given condition is met. Here’s an example in plain English: “If it’s snowing outside, I’ll need to shovel the sidewalk.” More precisely, “If the snow depth is greater than two inches, I’ll need to shovel.” Even more precisely, my wife will need to shovel (she likes to); however, I won’t try to represent that final case programmatically. In JavaScript, this snow depth condition might be written as such:
if (snowDepth > 2) { goShovel(); }
The syntax for an if conditional calls for the test to be placed in parentheses and the code to be executed within braces, as in the preceding example. This construct is similar to the loop construct you saw in the previous section.
You can also use conditionals to define an “otherwise” scenario. Going back to the plain-English example: “If the snow depth is greater than two inches, go shovel; otherwise, watch the game.” In code, you can represent this scenario with an else statement:
if (snowDepth > 2) { goShovel(); } else { enjoyGame(); }
You aren’t limited to evaluating single conditions; you can evaluate multiple condition scenarios, as well. For example, using the snow example one last time (I promise), I might like to go skiing if there is more than 10 inches of snow. This is represented in code using the else if statement, as shown here:
if (snowDepth > 10) { goSkiing(); } else if (snowDepth > 2) { goShovel(); } else { enjoyGame(); }
Note that the order of these conditionals is vital. For example, if I test whether the snowDepth is greater than 2 inches first, the code that checks whether the snowDepth is 10 inches would never be executed because snow that’s 10 inches is also greater than 2 inches.
Conditions can also be combined into one set of tests, either logically together or as an either-or scenario. Here are some examples:
if (firstName == "Steve" || firstName == "Jakob") { alert("hi"); }
In this example, if the variable firstName is set to either Steve or Jakob, the code will execute. This code uses the logical OR syntax, represented by two pipe characters (||). Conditions can be joined with the logical AND syntax, represented by two ampersands (&&), as in this example:
if (firstName == "Steve" && lastName == "Suehring") { alert("hi"); }
In this example, if firstName is Steve and the lastName is set to Suehring, the code will execute.
A Conditional Example
Earlier in the chapter, you learned about two types of equality operators: the double-equal sign (==) and the triple-equal sign (===). The triple-equal sign operator tests not only for value equality, but it also checks that each value is the same type. As promised, here’s a more complete example. To try this example, use the sample page and external JavaScript file you created earlier in this chapter. This code can be found within the file cond.html in the companion content.
Within the external.js JavaScript file, place the following code, replacing any code already in the file:
var num = 42.0; var str = "42"; if (num === str) { alert("num and str are the same, even the same type!"); } else if (num == str) { alert("num and str are sort of the same, value-wise at least"); } else { alert('num and str are different'); }
Save that file, and run the project in Visual Studio (press F5). An alert appears, such as the one shown in Figure 2-10. Note that you should be viewing the index.html page, because that’s the location from which external.js is referenced.

Figure 2-10 Testing equality operators with a string and number.
Now remove the quotes from the str variable, so that it looks like this:
var str = 42;
View the page again, and you’ll get an alert like the one shown in Figure 2-11.

Figure 2-11 Evaluating two numbers in JavaScript, using the strict equality test.
This second example illustrates a nuance of JavaScript that might not be apparent if you’ve programmed in another language. Notice that the num variable is actually a floating-point number, 42.0, whereas the str variable now holds an integer. As previously stated, JavaScript doesn’t have separate types for integers and floating-point numbers; therefore, this test shows that 42.0 and 42 are the same.
As a final test, change the num variable to this:
var num = 42.1;
View the page one final time. An alert similar to the one shown in Figure 2-12 appears.
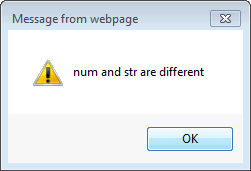
Figure 2-12 Testing equality with different values.
This final example showed not only how to combine conditional tests, but also how the equality operators work in JavaScript.