JavaScript Programming Basics
- 9/15/2012
- JavaScript Placement: Revisited
- Basic JavaScript Syntax
- JavaScript Variables and Data Types
- Looping and Conditionals in JavaScript
- Summary
JavaScript Variables and Data Types
Variables and data types define how a programming language works with user information to do something useful. This section will look at how JavaScript defines variables and the data types within the language.
Variables
Variables contain data that might change during the course of a program’s lifetime. Variables are declared with the var keyword. You’ve seen several examples of this throughout the book already, but here are a few more:
var x = 19248; var sentence = "This is a sentence."; var y, variable2, newVariable;
Variable values are set or initialized by using the equals sign (=) or equals operator.
Two items are used in a similar fashion to variables: arrays and objects. Objects are discussed in the next chapter. In JavaScript, arrays are actually an object that acts like an array. For our purposes, we’ll treat them as standard arrays, though, because the differences aren’t important at this stage.
But wait, what’s an array? Arrays are collections of items arranged by a numerical index. Put another way, think of the email messages in your inbox. If you’re like me, that inbox is stacked with hundreds of emails. In programming terms, this is an array of emails. The emails in my inbox begin at 1 and continue through 163. In programming, however, it’s typical for arrays to start at the number 0 and continue on—so rather than having emails 1 through 163, I’d have 0 through 162. When I want to access an item in the email inbox array, I would do so by using its numbered index.
In JavaScript, arrays are created with something called the array literal notation, [], like this:
var myArray = [];
That syntax indicates that a variable called myArray will be an array. The line of code shown merely declares that this variable will be an array instead of a string or number, sort of like an empty email inbox.
Rather than declaring an empty array, you can also populate an array as you create it, such as in this example that creates an array containing the types of trees outside of my house:
var myArray = ['Pine','Birch','Maple','Oak','Elm'];
Arrays can contain values of any type, such as numbers and strings, mixed within the array, as in this example:
var anotherArray = [32.4,"A string value",4, -98, "Another string!"];
Arrays have a special property, length, that returns the length of the array. In JavaScript, the length represents the number of the final index that has been defined, which might or might not be the same as the number of elements defined. This calls for an example! This example uses the sample page created earlier in this chapter, along with the external JavaScript file created earlier.
Within the external JavaScript file, place the following code:
var myArray = ['Pine','Birch','Maple','Oak','Elm']; alert(myArray.length);
The external.js JavaScript file should look like Figure 2-4.
Now, view the page (index.html) in a browser. In Visual Studio, on the Debug menu, click Start Debugging, or press F5. Your browser opens and an alert appears, such as that shown in Figure 2-5, containing the length of the array.
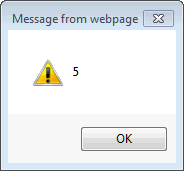
Figure 2-5 The length of the myArray array, thanks to the length property.
Now, replace the code in external.js with this code:
var myArray = []; myArray[18] = "Whatever"; alert(myArray.length);
Running this script yields an alert like the one shown in Figure 2-6.
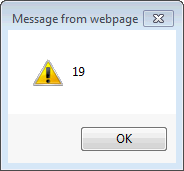
Figure 2-6 The alert shows that the length of myArray is 19.
The alert shows a length of 19 even though only one element was defined. Therefore, be aware when using the length property that the number returned might not be the true length of all of the elements in the array.
Also, note that the example defined index number 18 of the array but the length returned was 19. Remember, array indexes begin with 0 not 1, so index 18 is really the 19th element in the array, thus the length of 19.
JavaScript defines several methods for working with arrays. Some highlights are shown in Table 2-2. They will be discussed further as they are used in the book.
Table 2-2 Methods for working with arrays in JavaScript
Method |
Description |
concat |
Joins two arrays together or appends additional items to an array, creating a new array. |
join |
Creates a string from the values in an array. |
pop |
Removes and returns the last element of an array. |
push |
Adds or appends an element to an array. |
reverse |
Changes the order of the elements in the array so that the elements are backwards. If the elements were a, b, c, they will be c, b, a after the use of reverse. |
shift |
Removes the first element from the array and returns it. |
sort |
Sorts elements of an array. Note that this method assumes that elements are strings, so it won’t sort numbers correctly. |
unshift |
Places an item or items at the beginning of an array. |
Additionally, later in this chapter you’ll see how to cycle through each of the elements in an array using looping constructs in JavaScript.
Data Types
The data types of a language are some of the basic elements or building blocks that can be used within the program. Depending on your definition of data type, JavaScript has either three or six data types. The main data types in JavaScript include numbers, strings, and Booleans, with three others, null, undefined, and objects, being special data types. We’ll discuss most of these data types here and leave the discussion of objects for Chapter 3.
Numbers
There is one number type in JavaScript, and it can be used to represent both floating-point and integer values (1.0 and 1, respectively). Additionally, numbers in JavaScript can hold negative values with the addition of the minus (–) operator, as in –1 or –54.23.
JavaScript has built-in functions or methods for working with numbers. Many of these are accessed through the Math object, which is discussed in Chapter 3. However, one handy function for working with numbers is the isNaN() function. The isNaN() function—an abbreviation for “Is Not a Number”—is used to determine whether a value is a number. This function is helpful when validating user input to determine if a number was entered. Consider this example:
var userInput = 85713; alert(isNan(userInput));
In this example, the output will be false, because 85713 is a number. It does take a bit of mental yoga to think in terms of the negative “not a number” when using this function. It would have been better for the function to be defined in the affirmative, as in “Is this a number?”
Strings
Strings are sequences of characters (one or more) enclosed in quotation marks. The following are examples of strings:
"Hello world" "B" "Another 'quotable string'"
The last example bears some explanation. Strings can be quoted with either single or double quotation marks in JavaScript. When you need to include a quoted string within a string, as in the example, you should use the opposite type of quotation mark. In the example, double quotes were used to enclose the string, whereas single quotes were used to encapsulate the quoted string within.
You can also use an escape sequence or escape character to use quotation marks within the same type of quotation marks. The backslash (\) character is used for this purpose, as in this example:
'I\'m using single quotes within this example because they\'re common characters in the text.'
Other escape sequences are shown in Table 2-3.
Table 2-3 Escape sequences in JavaScript
Escape Character |
Sequence Value |
\b |
Backspace |
\t |
Tab |
\n |
Newline |
\v |
Vertical tab |
\f |
Form feed |
\r |
Carriage return |
\\ |
Literal backslash |
Strings have methods and properties to assist in their use. The length property provides the length of a string. Here’s a simple example:
"Test".length;
This example returns 4, the length of the word Test. The length property can also be used on variables, as in this example:
var myString = "Test String"; myString.length; // returns 10
Strings include several other methods, including toUpperCase and toLowerCase, which convert a string to all uppercase or lowercase, respectively. Additionally, JavaScript allows concatenation or joining together strings and other types by using a plus sign (+). Joining a string can be as simple as this:
var newString = "First String, " + "Second String";
The preceding line of code would produce a variable containing the string “First String, Second String”. You’ll see this type of concatenation throughout the book.
Other methods for changing strings include charAt, indexOf, substring, substr, and split. Some of these methods will be used throughout the remainder of this book and will be explained in depth as they are used.
Booleans
Booleans have only two values, true and false, but you don’t work with Booleans in the same way that you work with other variables. You can’t create a Boolean variable, but you can set a variable to true or false. You’ll typically use Boolean types within conditional statements (if/else) and as flags to test whether something is true or false.
An important point to remember with Booleans is that they are their own special type. When setting a variable to a Boolean, you leave the quotes off, like this:
var myValue = true;
This is wholly different than setting a variable to a string containing the word “true”—in which case, you include the quotes:
var myValue = "true";
Let’s test that scenario with a code sample. The example uses the index.html page shown earlier, along with the external JavaScript file, external.js, created in this chapter. Remove any existing code from within the external.js file and replace it with this code:
var myString = "true"; var myBool = true; alert("myString is a " + typeof(myString)); alert("myBool is a " + typeof(myBool));
Save external.js. It should look like the example in Figure 2-7.
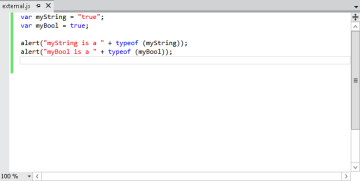
Figure 2-7 The external.js file should contain this JavaScript.
No changes are necessary to the index.html file because external.js is already referenced in that file. Now view index.html. In Visual Studio, on the Debug menu, click Start Debugging, or press F5. Two alert dialogs boxes appear, like those shown in Figures Figure 2-8 and Figure 2-9. You can find the code for this example in bool.html and bool.js in the companion content.
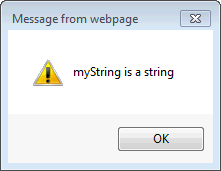
Figure 2-8 The variable myString is a string value, even though the string is set to the word true.
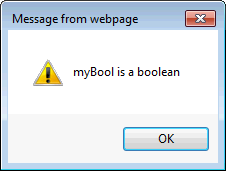
Figure 2-9 The variable myBool is a true Boolean value.
This difference between the string “true” and the Boolean value true can be important when performing conditional tests. You’ll learn more about conditional tests later in this chapter.
Null
Null is a special data type in JavaScript. Null is nothing; it represents and evaluates to false, but null is distinctly different than empty, undefined, or Boolean. A variable can be undefined (you haven’t initialized it yet, for example), or a variable can be empty (you set it to an empty string, for example). Both are different from null.
Undefined
Undefined is a type that represents a variable or other element that doesn’t contain a value or has not yet been initialized. This type can be important when trying to determine whether a variable exists or still remains to be set. You’ll see examples of undefined throughout the book.