Hypertext Markup Language (HTML)
- By Rob Miles
- 9/10/2021
Egg timer
We now know enough to be able to create a properly useful program. We are going to create an egg timer. The user will press a button and then be told when five minutes (the perfect time for a boiled egg) have elapsed. We know how to connect a JavaScript function to an HTML button. The next thing we need to know is how to measure the passage of time. We can do this by using a JavaScript function called setTimeout. We have used functions already. The alert function accepts a string that it displays. The setTimeout function accepts two things: a function that will be called when the timer expires and the length of the timeout. The timeout length is given in thousandths of a second. The statement below will cause the doEndTimer function to run one second after setTimout was called.
setTimeout(doEndTimer,1000);
Our egg timer will use two functions. One function will run when the user presses a button to start the timer. This function will set a timer that will run the second function after five minutes. The second function will display an alert that indicates that the timer has completed.
function doStartTimer() { setTimeout(doEndTimer,5*60*1000); } function doEndTimer() { alert("Your egg is ready"); }
The doStartTimer function is connected to a button so that the user can start the timer. The doEndTimer will be called when the timer completes. I’ve added a calculation that works out the delay value. I want a five-minute delay. There are 60 seconds in a minute and a value of 1000 would give me a one-second delay. This makes it easier to change the delay. If we want to make a hard-boiled egg that takes seven minutes, I just have to change the 5 to a 7. Note that the * character is used in JavaScript to mean multiply. You will find out more about doing calculations in Chapter 4.
Adding sound to the egg timer
Our egg timer works fine, but it would be nice if it could do a little more than just display an alert when our egg is ready. A web page can contain an audio element that can be used to play sounds.
<audio id="alarmAudio"> <source src="everythingSound.mp3" type="audio/mpeg"> Your browser does not support the audio element. </audio>
The audio element includes another element called src that specifies where the audio data is going to come from. In this case, the audio is stored in an MP3 file named everythingSound.mp3, which is stored on the server. The text inside the audio element is displayed if the browser does not support the audio element. I’ve given this element an ID so that the code in the doEndTimer function can find the audio element and ask it to play the MP3 file.
function doEndTimer() { alarmSoundElement = document.getElementById("alarmAudio"); alarmSoundElement.play(); }
This code looks like the code in the Ch02-08 Paragraph Update example. In that case, the getElementById method was fetching a paragraph element to be updated. In the function above, getElementById is fetching an audio element to be played. An audio element provides a play method that starts it playing. The rest of the file is exactly the same as the original egg timer. If you try this program, you will hear quite an impressive sound when your egg is ready.
Controlling audio playback
The egg timer page does not display anything to represent the audio element. It is “hidden” inside the HTML. You can modify an audio element so that a player control is shown on the web page. To do this, you just have to add the word controls into the element tag:
<!DOCTYPE html> <html lang="en"> <head> <title>Ch02-11 Sound playback</title> </head> <body> <audio controls> <source src="everythingSound.mp3" type="audio/mpeg"> Your browser does not support the audio element. </audio> </body> </html>
This is the complete source of an MP3 file playback page. If you visit the page you will see a simple playback control, as shown in Figure 2-13.
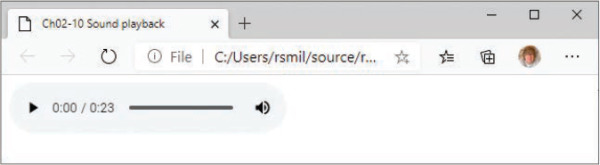
FIGURE 2-13 Sound playback
This is how the playback control looks when using the Edge browser. Other browsers will look slightly different, but the fundamental controls will be the same. You can start the playback by pressing the play control at the far left.