Programming in HTML5 with JavaScript and CSS3: Access and Secure Data
- 8/25/2014
- Objective 3.1: Validate user input by using HTML5 elements
- Objective 3.2: Validate user input by using JavaScript
- Objective 3.3: Consume data
- Objective 3.4: Serialize, deserialize, and transmit data
- Answers
Most web applications require static or dynamic data. Static data is written directly into the HTML markup, not altered or loaded by code such as JavaScript. It’s rendered and displayed to users without any way for the data to change. Dynamic data can change. Dynamic data can update a ticker on a webpage from a news feed, capture user data to perform an operation and provide results, or perhaps even store just a user’s registration information in a database.
Both approaches to data have benefits as well as disadvantages. Static data is quite secure because it doesn’t provide much of an attack surface for a malicious user. However, as a website transitions into a more dynamic site, with live updates of data and the ability for users to enter information into various fields, an attack surface opens and the site can become less secure.
Knowing how to prevent malicious users from causing harm to your application and possibly your users is important. You can implement the same mechanisms used to prevent malicious usage to simplify the user experience and to keep your data generally clean. Certain data elements, such as phone numbers and email addresses, can be provided in different formats. Because such information can be very important, you want to make it as easy as possible for users to enter it. Having complete address information and ensuring that all the necessary fields are populated also can be very important. HTML5 supports constructs such as regular expressions and required attributes to support implementing these types of rules. Throughout the objectives in this chapter, validating user input both declaratively via HTML5 and also by using JavaScript is covered.
In other scenarios, data coming to and from the website is either consuming data feeds or providing data to another destination. Websites today commonly have a direct link to social networking updates. In these cases, the retrieving and sending of the data is invisible in that users aren’t engaged with the process. These processes should be streamlined and not interfere with the website’s user experience. In this chapter’s objectives, consuming data from external sources, transmitting data, and serializing and deserializing data are all covered.
Objectives in this chapter:
- Objective 3.1: Validate user input by using HTML5 elements
- Objective 3.2: Validate user input by using JavaScript
- Objective 3.3: Consume data
- Objective 3.4: Serialize, deserialize, and transmit data
Objective 3.1: Validate user input by using HTML5 elements
This objective examines the user interface elements made available by HTML5 that allow users to provide input. The ability to capture information from users is a great feature. However, you must ensure that user privacy and safety are protected as best as possible. You also must ensure that the website doesn’t open any holes that an attacker can exploit to disrupt the site’s services. Part of protecting the site is choosing the correct user input controls for the job and setting the appropriate attributes on those controls to ensure that the data is validated. For the exam, you need to know these input controls and the attributes they use for this purpose.
Choosing input controls
HTML5 provides a wide assortment of controls to make capturing user input simple and secure. In this section, you explore the user input controls in greater detail and see examples of their usage. A simulation of a survey form will be created to demonstrate when each type of control should be used. Listing 3-1 shows the entire markup for the survey.
LISTING 3-1 HTML5 markup for a customer survey,
<form> <div> <hgroup> <h1>Customer Satisfaction is #1</h1> <h2>Please take the time to fill out the following survey</h2> </hgroup> </div> <table> <tr> <td>Your Secret Code: </td> <td> <input type="text" readonly="readonly" value="00XY998BB"/> </td> </tr> <tr> <td>Password: </td> <td> <input type="password"/> </td> </tr> <tr> <td>First Name: </td> <td> <input type="text" id="firstNameText" maxlength="50"/> </td> </tr> <tr> <td>Last Name: </td> <td> <input type="text" id="lastNameText"/> </td> </tr> <tr> <td> Your favorite website: </td> <td> <input type="url"/> </td> </tr> <tr> <td> Your age in years: </td> <td> <input type="number"/></td> </tr> <tr> <td> What colors have you colored your hair: </td> <td> <input type="checkbox" id="chkBrown" checked="checked"/> Brown <input type="checkbox" id="chkBlonde"/> Blonde <input type="checkbox" id="chkBlack"/> Black <input type="checkbox" id="chkRed"/> Red <input type="checkbox" id="chkNone"/> None </td> </tr> <tr> <td>Rate your experience: </td> <td> <input type="radio" id="chkOne" name="experience"/> 1 - Very Poor <input type="radio" id="chkTwo" name="experience"/> 2 <input type="radio" id="chkThree" name="experience"/> 3 <input type="radio" id="chkFour" name="experience"/> 4 <input type="radio" id="chkFive" name="experience" checked="checked"/> 5 - Very Good </td> </tr> <tr> <td>How likely would you recommend the product: </td> <td> <br/> <br/> <br/> <br/> <input type="range" min="1" max="25" value="20"/> </td> </tr> <tr> <td> Other Comments: </td> <td> <textarea id="otherCommentsText" rows="5" cols="20" spellcheck="true"> </textarea> </td> </tr> <tr> <td> Email address: </td> <td> <input type="email" placeholder="me@mydomain.com" required/> </td> </tr> <tr> <td> <input type="submit"/> <input type="reset"/> <input type="button" value="Cancel"/> </td> </tr> </table> </form>
The <input> element in HTML denotes input controls. This element contains a type attribute that specifies the type of input element to render. The exceptions to the <input type=’’> rule are the <textarea> and <button> elements, which have their own element support. Table 3-1 outlines the input elements supported in HTML5 and indicates whether an element is now supported in Internet Explorer. The additional attributes available to an <input> element are discussed in later sections.
TABLE 3-1 HTML5 input elements
Element |
Description |
color* |
Provides a color picker |
date* |
Provides a date picker |
datetime* |
Provides a date/time picker |
month* |
Enables users to select a numeric month and year |
week* |
Enables users to select a numeric week and year |
time* |
Enables users to select a time of day |
number* |
Forces the input to be numeric |
Range |
Allows users to select a value within a range by using a slider bar |
tel* |
Formats entered data as a phone number |
url |
Formats entered data as a properly formatted URL |
Radio† |
Enables users to select a single value for a list of choices |
Checkbox† |
Enables users to select multiple values in a list of choices |
Password† |
Captures a password and glyphs the entered characters |
Button† |
Enables users to perform an action such as run script |
Reset† |
Resets all HTML elements within a form |
Submit† |
Posts the form data to a destination for further processing |
Using text and textarea input types
The text and textarea input controls are the most flexible. By using these controls, you allow users to enter any text that they want into a regular text box. A text box provides a single-line text entry, whereas a textarea allows for a multiline data entry. The following HTML shows the markup for both types of controls:
<table> <tr> <td> First Name: </td> <td> <input type="text" id="firstNameText"/> </td> </tr> <tr> <td> Last Name: </td> <td> <input type="text" id="lastNameText"/> </td> </tr> ... <tr> <td>Other Comments: </td> <td> <textarea id="otherCommentsText" rows="5" cols="20"></textarea> </td> </tr> </table>
Figure 3-1 shows the output of this code.

FIGURE 3-1 HTML markup showing text box data-entry fields
This code adds text boxes to capture information such as first name, last name, and additional comments. For the first and last names, the input is a standard text box as denoted by type=“text”. This tells the renderer to display an input field into which users can enter free-form text. However, this type of input field is limited to a single line. The Other Comments text box provides a multiline text area for users to enter text into. The rows and cols attributes define the viewable size of the text area. In this case, users can enter many lines of text into the text area.
Other attributes that help with controlling how much information is entered into the text fields is the maxlength attribute:
<input type="text" id="firstNameText" maxlength="50"/>
Users can’t enter any more than 50 characters into the text field with the maxlength set to a value of 50.
In some cases, you might want to ensure that users enter only certain information in a certain format.
url input type
The <input> type of url displays a text box similar to what the <input> type of text provides. However, the renderer is instructed that the input type is url, so when users try to submit a form with this type of information on it, it validates that the text in the box matches the format of a valid URL.
The following code demonstrates a url type added to the survey:
<tr> <td>Last Name: </td> <td> <input type="text" id="lastNameText"/> </td> </tr> <tr> <td> Your favorite website: </td> <td> <input type="url"/> </td> </tr> <tr> <td> Other Comments: </td> <td> <textarea id="otherCommentsText" rows="5" cols="20"></textarea> </td> </tr> ... <tr> <td> <input type="submit"/> </td> </tr>
This code produces the output shown in Figure 3-2 to the HTML page making up the survey. This HTML code also adds an input button, as discussed later in the section, “Using the button input type.”
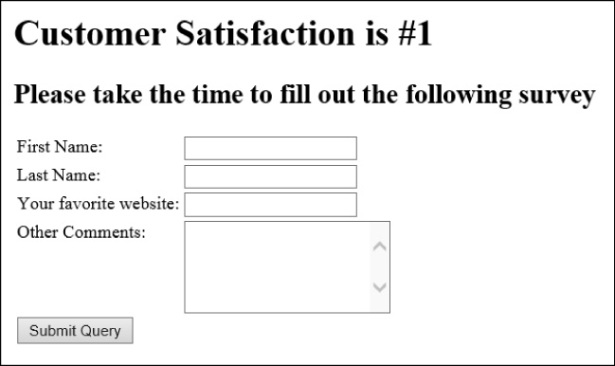
FIGURE 3-2 The url input box added to the survey
This code demonstrates the power of the url input type in validating that the text a user entered is indeed a valid URL format. If a user typed something other than a URL or an incomplete URL into the Your Favorite Website box, such as contoso.com, and then clicked the Submit Query button, the result would be similar to the output shown in Figure 3-3.
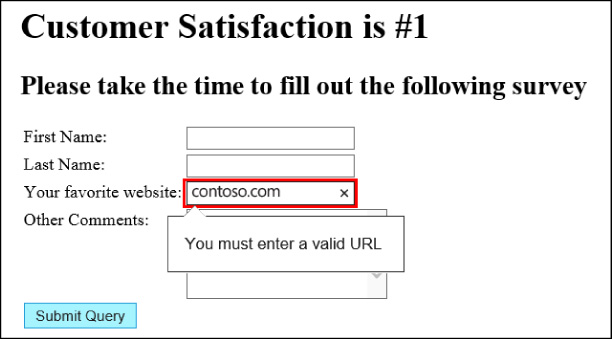
FIGURE 3-3 Demonstrating the validation of the url input type
Click the button to invoke the validation. The url box is outlined in red, and a tooltip pops up to explain the validation error. In this case, it has detected that a valid URL hasn’t been entered. If the user corrects the data by specifying the URL as http://www.contoso.com, the validation error doesn’t occur and the input can be submitted successfully.
If you require more flexibility and want to accept partially entered URL information, such as contoso.com, don’t use the url input box. A regular text input with a pattern specified would be more appropriate.
Using the password input control
The password input control is the standard method of prompting users for sensitive information. As you type your password, each character is replaced with a glyph so that any onlookers can’t see your password.
You can add a password text box to the survey to provide a way to retrieve a survey if a user wants to complete it later. The password could be stored in a server for later retrieval. The following markup is added to the HTML:
<tr> <td> Password: </td> <td> <input type="password"/> </td> </tr>
With this HTML added, the survey now appears as shown in Figure 3-4.
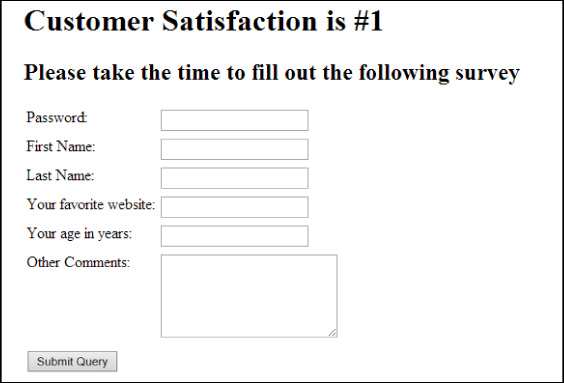
FIGURE 3-4 A password input field added to the form
Again, the password text box doesn’t look any different than any other text box. However, typing into the box provides a different experience, as shown in Figure 3-5.

FIGURE 3-5 Replacing password input with the glyph character
The password input type captures information securely. Users typing this information don’t want others who are nearby to be able to see what they’ve been typing and hence compromise their data.
Using the email input type
You can use the email input type to ensure that the format of the text entered into the text box matches that of a valid email address. Being able to capture an email address is often important to enable further follow up with a user. This control helps ensure that the information entered matches what’s expected in the form of an email address.
The following HTML adds an email address input type to the survey:
<tr> <td> Email address: </td> <td> <input type="email"/> </td> </tr> <tr> <td> <input type="submit"/> </td> </tr>
Figure 3-6 shows the output of this HTML.

FIGURE 3-6 Output of the email address input type
Just as with the url input type, if you type text that doesn’t match the format of an email address, you receive a warning message (see Figure 3-7).

FIGURE 3-7 Validation for the email address input type
This validation helps ensure that you don’t mistype your email address. Of course, it doesn’t prevent you from entering an invalid email address, only one where the format doesn’t match correctly to what would be expected such as having the @ symbol and ending with a .com or other domain suffix.
Using the checkbox input type
In some cases when capturing information from users, you need to be able to capture more than one choice as it relates to a specific question. In this case, the checkbox input control is the best choice. You can provide a series of check boxes and allow users to select all that apply.
The survey will now add a question where users can select multiple items, as follows:
<tr> <td>Your age in years:</td> <td><input type="number" /></td> </tr> <tr> <td> What colors have you colored your hair: </td> <td> <input type="checkbox" id="chkBrown"/> Brown <input type="checkbox" id="chkBlonde"/> Blonde <input type="checkbox" id="chkBlack"/> Black <input type="checkbox" id="chkRed"/> Red <input type="checkbox" id="chkNone"/> None </td> </tr>
In this HTML example, users see a list of hair colors that they might have used. Because a user possibly might have used more than one, she has the option to choose more than one. Figure 3-8 shows the output of this HTML.
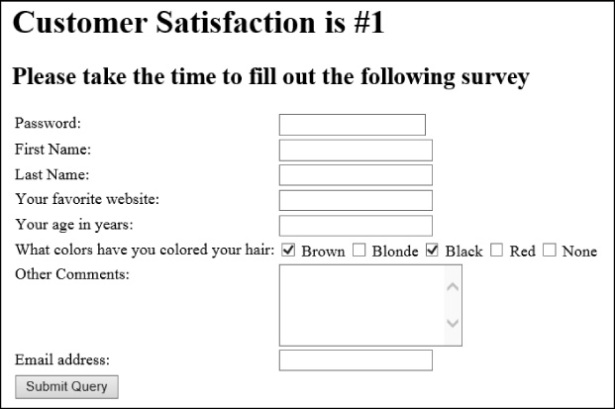
FIGURE 3-8 The input check box added to the HTML form
An additional attribute available on the check box is the checked attribute. This attribute provides a way to default a check box to the “checked” (or selected) state. By default, check boxes aren’t selected. However, by adding the attribute as follows, the check box defaults to the “checked” state when the page is loaded:
<input type="checkbox" id="chkBrown" checked="checked"/> Brown
In other cases, when presented with a list of items, users might be able to choose only a single item from the list.
Using the radio input type
The radio button is similar to the check box in that it provides a list of options for users to select from. The difference from the check box is that users can select only a single item from the list. An example would be asking users to rate something on a scale from 1 to 5. To add this type of question to the survey, incorporate the following HTML beneath the check boxes:
<tr> <td> Rate your experience: </td> <td> <input type="radio" id="chkOne" name="experience"/> 1 - Very Poor <input type="radio" id="chkTwo" name="experience"/> 2 <input type="radio" id="chkThree" name="experience"/> 3 <input type="radio" id="chkFour" name="experience"/> 4 <input type="radio" id="chkFive" name="experience"/> 5 - Very Good </td> </tr>
Notice that as with all HTML elements, each radio input type needs a unique id. However, the name attribute ties all the radio buttons together. With the same name specified for each radio type, the browser knows that they are part of a group and that only one radio button of the group can be selected. Figure 3-9 shows the output of the radio buttons added to the survey.

FIGURE 3-9 Adding some radio input types to the form
In this output, the radio buttons are shown from left to right and enable users to select only one option. When a user changes the selection to a different option, the previously selected option is automatically cleared.
Like with the checkbox input types, defaulting the state of the radio input to selected is possible. This is done in exactly the same way, by specifying the checked attribute:
<input type="radio" id="chkFive" name="experience" checked="checked"/> 5 - Very Good
In this case, the rating of 5 - Very Good defaults to selected for the group of radio buttons.
You can have multiple groups of radio buttons on the same page by specifying a different name for each group of buttons. Another way to provide users with the ability to specify a single value within a group of values is with the use of the range control.
Using the range input type
Using the range input type enables users to specify a value within a predefined range by using a slider bar. This type can be used in cases where a wider range of values is required to choose from but using radio buttons would be too unwieldy. Add another rating question to the survey, as shown in the following HTML after the radio buttons:
<tr> <td>How likely would you recommend the product: </td> <td> <input type="range" min="1" max="25" value="20"/> </td> </tr>
This HTML markup provides users with a slider bar that they can use to specify a value between 1 and 25. The min attribute specifies the minimum value of the range; the max attribute specifies the maximum value. The value attribute specifies a default value. If you omit the value attribute, the range defaults to the minimum value. This HTML displays the output shown in Figure 3-10.
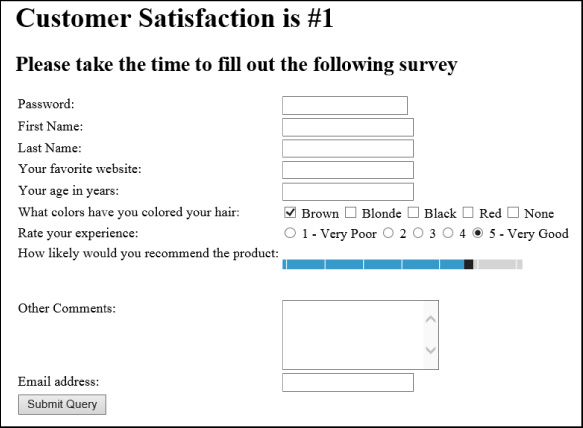
FIGURE 3-10 A range input element added to the HTML form
In this output, the range control is displayed as a slider bar. The bar defaults to the value of 20 as specified in the markup. Users can grab the black endpoint of the slider and change the value lower or higher by dragging it left or right. As a user changes the value, a tooltip shows the current value where the slider resides. In this case, the user is now at the value 17 (see Figure 3-11).

FIGURE 3-11 The tooltip displaying the current value of the range as the user changes it.
After users enter all the needed information, they need a way to submit or save the information. The submit button has already been previewed.
Using the button input type
The input type that allows users to submit the form or clear it is button. The button input isn’t new to HTML5 but is an essential piece to the data-capture puzzle. Buttons are what tell the website when a user finishes doing something and that they want to perform an action. The <input> element supports three types of button controls: submit, reset, and button.
The submit input type tells the HTML form to post its information to the server (or, in some cases, to another site or webpage). The reset type automatically clears all form elements to their default values. The button type provides a generic button with no predefined functionality. It can be used to provide a custom function, such as cancel out from this page and return to the home page. All three button types are added to the bottom of the survey page as follows:
<tr> <td> <input type="submit"/> <input type="reset"/> <input type="button"/> </td> </tr>
That’s all that’s required to add the functionality to the page for each button. Of course, type=“button” requires some JavaScript to be wired up to actually do something. However, the submit and reset buttons come with the described functionality built in. The HTML provides the output on the form as shown in Figure 3-12.

FIGURE 3-12 Buttons added to the HTML form
The text on the buttons is the default text. The submit button comes with the text Submit Query, and the reset button comes with the text Reset. This can’t be changed. However, the button type doesn’t have any text on it because none was specified and the button doesn’t come with any predetermined behavior. To specify text for this button, add the value attribute:
<input type="button" value="Cancel"/>
This produces a button as shown in Figure 3-13.

FIGURE 3-13 The button type with text specified
That’s what you get with the input type of button. However, in some cases, more flexibility in the button’s content is desired. This is where the button element comes in handy.
Using the button element
The button element provides a button on the user interface, just as the name implies. However, from a graphical perspective, this element behaves very differently.
The button element also supports a type attribute, like as the ones seen previously: submit, reset, and button. This example steps away from the survey and demonstrates these buttons on a stand-alone page. The following HTML is added to a page, and the subsequent output is shown in Figure 3-14:
<button type="button"/> <button type="reset"/> <button type="submit"/>

FIGURE 3-14 All three types of button elements
This output displays three buttons, as expected. However, it doesn’t provide any text on the buttons. The button element provides only the desired click behavior, such as submitting, resetting, or providing a custom behavior like with type=“button”. Everything else must be specified in the HTML, including the label or text that goes on the button. In this way, you have much more control over what’s put on the button. Instead of Submit Query as with the <input> element, the text can be set as Submit Survey or Save Data. The following HTML shows the text on the buttons, and Figure 3-15 shows the output:
<button type="button">Go Home</button> <button type="reset" >Reset</button> <button type="submit">Submit Survey</button>

FIGURE 3-15 The button elements with text specified
You can take the button element even further. The element’s contents don’t have to be just plain text. You can embed images within the element by using the <img> element in addition to text, or embed an entire clickable paragraph. You also can apply cascading style sheets (CSS) to the button to change its appearance, as shown in Figure 3-16. The HTML is as follows:
<button type="button" style="border-radius: 15px;"> <p>Something exciting lies behind this button</p> <img src=".\myimage.jpg"/> </button>

FIGURE 3-16 A customized button element
Within the button element lies the capability to create a highly customized button and get default behavior from the browser.
In addition to what’s provided by the various input types, such as range, email, and url, other attributes are available and common across most of the input controls and provide additional flexibility in how the fields are validated. This is covered next.
Implementing content attributes
Input controls provide content attributes that allow you to control their behavior in the browser declaratively rather than have to write JavaScript code.
Making controls read-only
Part of the specification for the HTML input controls includes a readonly attribute. If you want to present information to users in elements such as text boxes but don’t want them to be able to alter this data, use the readonly attribute. When readonly is specified, the renderer won’t allow users to change any of the data in the text box. The following HTML demonstrates the readonly property:
<tr> <td> Your Secret Code: </td> <td> <input type="text" readonly value="00XY998BB"/> </td> </tr>
In this code, at the top of the survey form, users are provided a secret code to correspond with their survey. They can’t change this because the readonly attribute is specified.
Where fields aren’t read-only and users can type whatever they want into the text box, providing them with the capability to check spelling is a good idea.
Providing a spelling checker
Checking spelling is another method available to validate user input. The spellcheck attribute helps provide feedback to users that a word they’ve entered is misspelled. Again, this attribute is applied to the input element:
<textarea id="otherCommentsText" rows="5" cols="20" spellcheck="true"></textarea>
In this HTML, the spellcheck option has been turned on for the Other Comments text area because users can type whatever they want and might make spelling errors.
The output of a text box with spellcheck isn’t any different until a user starts typing and enters a spelling error. Figure 3-17 shows the red underlining for the words that are detected as spelled incorrectly.

FIGURE 3-17 A textarea with spellcheck enabled
In some cases, the built-in validation provided by the input controls isn’t sufficient, and providing a custom pattern to validate is better, as explored in the next section.
Specifying a pattern
As you saw with the email and url input types, built-in validation is fairly thorough in ensuring that the information entered is accurate and as expected. However, in some cases you might require looser or stricter validation. Suppose that you don’t want users to have to specify the HTTP protocol in a url type, but you want to allow only .com or .ca websites. This can be achieved by using the pattern attribute, which allows the use of a regular expression to define the pattern that should be accepted.
The following code shows the pattern attribute used to achieve the desired validation:
<input type="text" title="Only .com and .ca are permitted." pattern="^[a-zA-Z0-9\-\.]+\.(com|ca)$"/>
Plenty of regular expressions are available to validate a URL; this one is fairly simple. When specifying the pattern attribute, you should specify the title attribute as well. The title attribute specifies the error message to users in the tooltip when validation fails.
To ensure that users enter the data in the correct format, you should show them a sample of what the data should look like. This is achieved with the placeholder attribute.
Using the placeholder attribute
The placeholder attribute enables you to prompt users with what’s expected in a certain text box. For example, an email text box might show placeholder text such as me@mydomain.com. More importantly, this placeholder text doesn’t interfere with users when they start typing their information into the text box. The placeholder attribute achieves this, as shown in the following HTML and subsequent output in Figure 3-18.
<input type="email" placeholder="me@mydomain.com" /></td>

FIGURE 3-18 The placeholder attribute demonstrating to users what is expected
The placeholder text is slightly lighter in color. As soon as a user puts the mouse cursor into the box to type, the placeholder text disappears and the user’s typing takes over.
HTML fields can be validated in many ways. In some cases, it’s not so much what is put into the field, but that the field is indeed filled in. The required attribute controls this for the HTML elements.
Making controls required
To ensure that a user fills in a field, use the required attribute with the <input> element. Doing so ensures that users will be told that the field is required. In this example, the email address will be made a required text box:
<input type="email" placeholder="me@mydomain.com" required/>
With the required control specified, if users try to submit the form without specifying an email address, they get an error message (see Figure 3-19). Now users can’t submit until they specify a valid email address.

FIGURE 3-19 The required field validation invoked
The capabilities of the input controls can provide quite a robust validation framework. However, more needs to be done to ensure that the website is safe and secure.
Objective summary
- Input controls such as text and textarea allow users to type information into a webpage.
- Some input controls provide built-in validation, such as for URLs and email addresses.
- Radio buttons and check boxes provide controls for users to select items in a list.
- Reset and submit buttons control behavior of the HTML form.
- Users can’t modify the content of a control that has the readonly attribute assigned.
- You can add a spelling checker to a text box to help users avoid spelling errors.
- The pattern attribute helps define a regular expression for custom validation of formatted data.
- The required attribute ensures that a field is populated before users can submit the form.
Objective review
Which input control is better suited for allowing users to make multiple selections?
- radio button
- textarea
- checkbox
- radio or checkbox
Which input control is designed to allow users to enter secure information in a way that keeps others from seeing what’s typed?
- text
- textarea
- url
- password
Which input control posts form data to a server?
- button
- Submit
- Reset
- radio
Which of the following declarations are valid ways to make a text control non-editable?
- <input type=“text” edit=“false”/>
- <input type=“text” editable=“false”/>
- <input type=“text” readonly=“yes”/>
- <input type=“text” readonly/>
How can you ensure that all necessary fields are populated before a form can be submitted?
- Write a JavaScript function to evaluate all the controls on the form for content.
- On the server, evaluate all the controls for data and return an error page for missing content.
- Add the required attribute on each control so that users get a message that the field is required.
- Add a label to the page to let users know which controls they must fill in.