What Is JavaScript?
- 9/15/2012
- A First JavaScript Program
- Where JavaScript Fits
- Writing Your First JavaScript Program
- Summary
After completing this chapter, you will be able to
Understand JavaScript’s role in a webpage
Create a simple webpage
Create a JavaScript program
WELCOME TO THE WORLD of JavaScript programming. This book provides an introduction to JavaScript programming both for the web and for Microsoft Windows 8. Like other books on JavaScript programming, this book shows the basics of how to create a program in JavaScript. However, unlike other introductory books on JavaScript, this book shows not only how something works but also why it works. If you’re looking merely to copy and paste JavaScript code into a webpage there are plenty of tutorials on the web to help solve those specific problems.
Beyond the basics of how and why things work as they do with JavaScript, the book also shows best practices for JavaScript programming and some of the real-world scenarios you’ll encounter as a JavaScript programmer.
Programming for the web is different than programming in other languages or for other platforms. The JavaScript programs you write will run on the visitor’s computer. This means that when programming for the web, you have absolutely no control over the environment within which your program will run.
While JavaScript has evolved over the years, not everyone’s computer has evolved along with it. The practical implication is that you need to account for the different computers and different situations on which your program might run. Your JavaScript program might find itself running on a computer from 1996 with Internet Explorer 5.5 through a dial-up modem just as easily as a shiny new computer running Internet Explorer 10 or the latest version of Firefox. Ultimately, this comes down to you, the JavaScript programmer, testing your programs in a bunch of different web browsers.
With that short introduction, it’s time to begin looking at JavaScript. The chapter begins with code. I’m doing this not to scare you away but to blatantly pander to the side of your brain that learns by seeing an example. After this short interlude, the chapter examines where JavaScript fits within the landscape of programming for the web and beyond. Then you’ll write your first JavaScript program.
A First JavaScript Program
Later in this chapter, you’ll see how to create your own program in JavaScript, but in the interest of getting you thinking about code right away, here’s a small webpage with an embedded JavaScript program:
<!doctype html> <html> <head> <title>Start Here</title> </head> <body> <script type="text/javascript"> document.write("<h1>Start Here!</h1>"); </script> </body> </html>
You’ll see later how to create a page like the one shown here. When viewed in a browser, the page looks like Figure 1-1. I’ll show you how to create such a page later in the chapter.
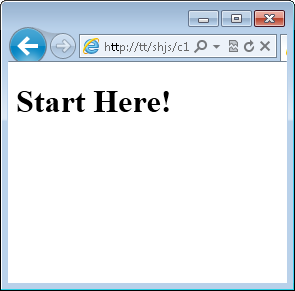
Figure 1-1 A basic JavaScript program to display content.
The bulk of the code shown in the preceding listing is standard HTML (HyperText Markup Language) and will be explained later. For now, you can safely ignore the code on the page except for the three lines beginning with <script type=“text/javascript”>.
The opening and closing script tags tell the web browser that the upcoming text is in the form of a script—in this case, it is of the type text/javascript. The browser sees that opening script tag and hands off processing to its internal JavaScript interpreter, which then executes the JavaScript. In this case, the entire code is merely contained on a single line: a call to the write method of the document object, which then places some HTML into the document.
The actual JavaScript comprises a single line:
document.write("<h1>Start Here!</h1>");
That line is enclosed within the opening and closing <script> tags. Each line of JavaScript is typically terminated by a semi-colon (;), and JavaScript gets executed from the top down. Each line is read in, then parsed and run, by the browser. The practical implication of the top-down execution is that if you have an error at the top, nothing below it will be executed by the browser. This can lead to some confusion when you’re expecting something to happen, like having words appear on the screen when in fact an error occurred near the top of the program that prevented the code from ever being run. Luckily, there are tools and techniques for troubleshooting JavaScript, which are discussed later on. The one exception to this top-down execution is in the area of functions, and you’ll see that later on, as well.
If you’re already feeling a bit lost, don’t worry. I’m going to back up and start from the beginning on JavaScript and describe its place on the web and among other programming languages.