Working with Functions in Windows PowerShell
- 11/11/2015
- Understanding functions
- Using functions to provide ease of code reuse
- Including functions in the Windows PowerShell environment
- Adding help for functions
- Using two input parameters
- Using a type constraint in a function
- Using more than two input parameters
- Using functions to encapsulate business logic
- Using functions to provide ease of modification
- Understanding filters
- Creating a function: Step-by-step exercises
- Chapter 6 quick reference
Adding help for functions
When you dot-source functions into the current Windows PowerShell console, one problem is introduced. Because you are not required to open the file that contains the function to use it, you might be unaware of everything the file contains within it. In addition to functions, the file could contain variables, aliases, Windows PowerShell drives, or any number of other things. Depending on what you are actually trying to accomplish, this might or might not be an issue. The need sometimes arises, however, to have access to help information about the features provided by the Windows PowerShell script.
Using a here-string object for help
In Windows PowerShell 1.0, you could solve this problem by adding a help parameter to the function and storing the help text within a here-string object. You can also use this approach in Windows PowerShell 5.0, but as shown in Chapter 7, “Creating advanced functions and modules,” there is a better approach to providing help for functions. The classic here-string approach for help is shown in the GetWmiClassesFunction.ps1 script, which follows. The first step that needs to be done is to define a switch parameter named $help. The second step involves creating and displaying the results of a here-string object that includes help information. The GetWmiClassesFunction.ps1 script is shown here.
GetWmiClassesFunction.ps1
Function Get-WmiClasses( $class=($paramMissing=$true), $ns="root\cimv2", [switch]$help ) { If($help) { $helpstring = @" NAME Get-WmiClasses SYNOPSIS Displays a list of WMI Classes based upon a search criteria SYNTAX Get-WmiClasses [[-class] [string]] [[-ns] [string]] [-help] EXAMPLE Get-WmiClasses -class disk -ns root\cimv2" This command finds wmi classes that contain the word disk. The classes returned are from the root\cimv2 namespace. "@ $helpString break #exits the function early } If($local:paramMissing) { throw "USAGE: Get-WmiClasses -class <class type> -ns <wmi namespace>" } #$local:paramMissing "`nClasses in $ns namespace ...." Get-WmiObject -namespace $ns -list | Where-Object { $_.name -match $class -and ` $_.name -notlike 'cim*' } # } #end get-wmiclasses
The here-string technique works pretty well for providing function help if you follow the cmdlet help pattern. This is shown in Figure 6-3.

FIGURE 6-3 Manually created help can mimic the look of core cmdlet help.
The drawback with manually creating help for a function is that it is tedious, and as a result, only the most important functions receive help information when you use this methodology. This is unfortunate, because it then requires the user to memorize the details of the function contract. One way to work around this is to use the Get-Content cmdlet to retrieve the code that was used to create the function. This is much easier than searching for the script that was used to create the function and opening it up in Notepad. To use the Get-Content cmdlet to display the contents of a function, you enter Get-Content and supply the path to the function. All functions available to the current Windows PowerShell environment are available via the Function Windows PowerShell drive. You can therefore use the following syntax to obtain the content of a function.
PS C:\> Get-Content Function:\Get-WmiClasses
The technique of using Get-Content to read the text of the function is shown in Figure 6-4.
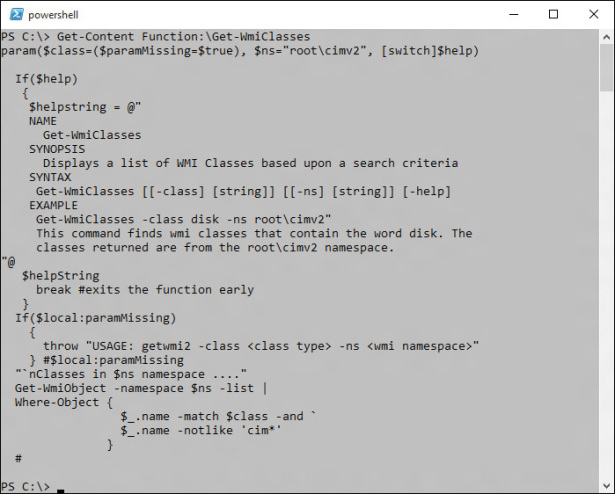
FIGURE 6-4 The Get-Content cmdlet can retrieve the contents of a function.
An easier way to add help, by using comment-based help, is discussed in Chapter 7. Comment-based help, although more complex than the method discussed here, offers a number of advantages—primarily due to the integration with the Windows PowerShell help subsystem. When you add comment-based help, users of your function can access your help in exactly the same manner as for any of the core Windows PowerShell cmdlets.